ceres最优估计方程规模计算
时间: 2024-04-25 15:21:03 浏览: 83
在Ceres Solver中,最优估计问题的规模可以通过参数数量和观测数量来计算。假设有$n$个参数和$m$个观测,那么问题的规模可以表示为$n+m$。
具体地,最优估计问题可以通过以下几个方程来描述:
1. 误差方程:假设我们有$m$个观测,每个观测都与一组参数相关。对于每个观测$i$,可以定义一个误差函数$f_i(\mathbf{x})$,其中$\mathbf{x}$是参数向量。这些误差函数描述了观测与参数之间的关系。
2. 最小二乘问题:我们的目标是最小化误差的平方和。将所有观测的误差函数平方和表示为一个目标函数:
$E(\mathbf{x}) = \sum_{i=1}^{m} f_i(\mathbf{x})^2$
这就是我们要优化的目标函数。
因此,对于最优估计问题,规模可以表示为$n+m$,其中$n$是参数的数量,$m$是观测的数量。
需要注意的是,规模只是衡量问题的大小,并不直接关联到求解的复杂性。复杂性还受到问题结构、初始估计、误差函数形式等因素的影响。在实际应用中,可以根据具体问题的规模和复杂性来选择适当的优化方法和策略。
相关问题
ceres实现最优估计算法,牛顿法、高斯牛顿法,阻尼最小二乘法 C++实现
Ceres是一款开源的C++库,用于实现非线性最小二乘问题的求解。它支持许多常见的最优化算法,包括牛顿法、高斯牛顿法和阻尼最小二乘法。
牛顿法是一种迭代法,用于寻找非线性函数的最小值。它使用函数的一阶和二阶导数来逼近函数,并使用这些信息来更新当前解的位置。Ceres实现了一种变形的牛顿法,称为LM(Levenberg-Marquardt)算法,它使用一个参数来平衡牛顿法和梯度下降法之间的权衡。
高斯牛顿法是一种迭代法,用于寻找非线性函数的最小值。它使用函数的一阶导数和雅克比矩阵来逼近函数,并使用这些信息来更新当前解的位置。Ceres实现了一种变形的高斯牛顿法,称为Schur-Jacobi算法,它使用一个参数来平衡高斯牛顿法和梯度下降法之间的权衡。
阻尼最小二乘法是一种迭代法,用于寻找非线性函数的最小值。它使用函数的一阶导数和雅克比矩阵来逼近函数,并使用这些信息来更新当前解的位置。不同于牛顿法和高斯牛顿法,阻尼最小二乘法在更新步长时引入了一个阻尼因子,以避免步长过大,从而导致求解过程不稳定。
下面是一个使用Ceres实现最小二乘问题的示例代码:
```c++
#include <iostream>
#include "ceres/ceres.h"
using namespace std;
// 定义残差函数
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(10.0) - x[0];
return true;
}
};
int main(int argc, char** argv) {
// 初始化问题
ceres::Problem problem;
// 添加变量
double x = 0.5;
problem.AddParameterBlock(&x, 1);
// 添加残差项
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor);
problem.AddResidualBlock(cost_function, nullptr, &x);
// 设置求解选项
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
options.minimizer_progress_to_stdout = true;
// 求解问题
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
// 输出结果
cout << summary.BriefReport() << endl;
cout << "x = " << x << endl;
return 0;
}
```
在这个例子中,我们定义了一个简单的残差函数,它的目标是让变量x的值接近10。然后,我们使用Ceres来求解这个最小二乘问题。我们首先将变量x添加到问题中,并添加一个残差项。然后,我们设置求解选项并调用ceres::Solve函数来求解问题。最后,我们输出求解结果。
这只是一个简单的例子,Ceres还支持更复杂的问题和算法。如果你想深入了解Ceres的使用,请参考官方文档。
ceres实现最优估计算法,C++分别实现牛顿法、高斯牛顿法,阻尼最小二乘法
以下是使用Ceres实现牛顿法、高斯牛顿法和阻尼最小二乘法的示例代码:
牛顿法:
```c++
#include <iostream>
#include "ceres/ceres.h"
using namespace std;
// 定义残差函数
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(10.0) - x[0];
return true;
}
};
int main(int argc, char** argv) {
// 初始化问题
ceres::Problem problem;
// 添加变量
double x = 0.5;
problem.AddParameterBlock(&x, 1);
// 添加残差项
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor);
problem.AddResidualBlock(cost_function, nullptr, &x);
// 设置求解选项
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
options.minimizer_progress_to_stdout = true;
options.trust_region_strategy_type = ceres::LEVENBERG_MARQUARDT;
// 求解问题
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
// 输出结果
cout << summary.BriefReport() << endl;
cout << "x = " << x << endl;
return 0;
}
```
高斯牛顿法:
```c++
#include <iostream>
#include "ceres/ceres.h"
using namespace std;
// 定义残差函数
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(10.0) - x[0];
return true;
}
};
int main(int argc, char** argv) {
// 初始化问题
ceres::Problem problem;
// 添加变量
double x = 0.5;
problem.AddParameterBlock(&x, 1);
// 添加残差项
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor);
problem.AddResidualBlock(cost_function, nullptr, &x);
// 设置求解选项
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
options.minimizer_progress_to_stdout = true;
options.trust_region_strategy_type = ceres::DOGLEG;
// 求解问题
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
// 输出结果
cout << summary.BriefReport() << endl;
cout << "x = " << x << endl;
return 0;
}
```
阻尼最小二乘法:
```c++
#include <iostream>
#include "ceres/ceres.h"
using namespace std;
// 定义残差函数
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(10.0) - x[0];
return true;
}
};
int main(int argc, char** argv) {
// 初始化问题
ceres::Problem problem;
// 添加变量
double x = 0.5;
problem.AddParameterBlock(&x, 1);
// 添加残差项
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor);
problem.AddResidualBlock(cost_function, nullptr, &x);
// 设置求解选项
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
options.minimizer_progress_to_stdout = true;
options.trust_region_strategy_type = ceres::DOGLEG;
options.dogleg_type = ceres::SUBSPACE_DOGLEG;
options.use_nonmonotonic_steps = true;
// 求解问题
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
// 输出结果
cout << summary.BriefReport() << endl;
cout << "x = " << x << endl;
return 0;
}
```
在这些示例中,我们将牛顿法、高斯牛顿法和阻尼最小二乘法的求解选项分别设置为LEVENBERG_MARQUARDT、DOGLEG和SUBSPACE_DOGLEG,并使用ceres::Solve函数求解问题。最后,我们输出求解结果。
阅读全文
相关推荐
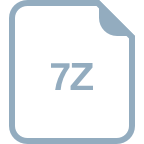
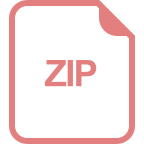
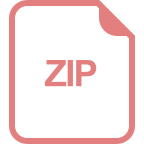
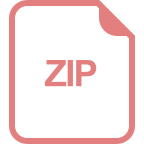
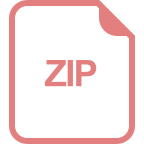
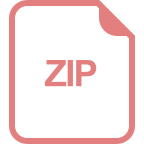
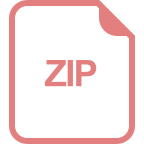
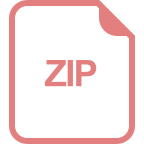
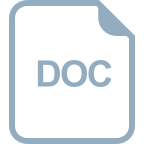







