ceres 代码实现牛顿法、高斯牛顿法,阻尼最小二乘法最优估计算法 C++
时间: 2023-06-23 19:50:10 浏览: 50
下面是Ceres中实现牛顿法、高斯牛顿法和阻尼最小二乘法的代码示例:
首先,我们定义一个继承自ceres::CostFunction的类,实现CostFunction::Evaluate方法。这里以一个简单的非线性最小二乘问题为例,目标是最小化函数f(x) = (x-2)^2。
```c++
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(x[0]) - T(2.0);
return true;
}
};
```
接着,我们定义一个ceres::Problem对象,并向其中添加CostFunction对象。
```c++
ceres::Problem problem;
double x = 0.5;
problem.AddResidualBlock(new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor), nullptr, &x);
```
然后,我们定义一个ceres::Solver::Options对象,设置优化器的参数,并调用ceres::Solve函数进行求解。
```c++
ceres::Solver::Options options;
options.minimizer_progress_to_stdout = true;
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
std::cout << summary.FullReport() << std::endl;
```
在Solve函数中,Ceres会自动选择合适的算法进行求解。如果需要指定某个特定的算法,可以在Solver::Options对象中设置相应的参数。例如,如果要使用牛顿法进行求解,可以将options.minimizer_type设置为ceres::TRUST_REGION_NEWTON。
下面是完整的代码示例:
```c++
#include <iostream>
#include "ceres/ceres.h"
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(x[0]) - T(2.0);
return true;
}
};
int main(int argc, char** argv) {
google::InitGoogleLogging(argv[0]);
double x = 0.5;
ceres::Problem problem;
problem.AddResidualBlock(new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor), nullptr, &x);
ceres::Solver::Options options;
options.minimizer_progress_to_stdout = true;
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
std::cout << summary.FullReport() << std::endl;
return 0;
}
```
相关推荐
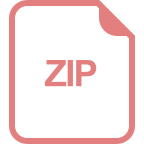
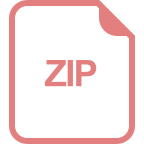














