ceres实现最优估计算法,C++分别实现牛顿法、高斯牛顿法,阻尼最小二乘法
时间: 2024-03-19 15:45:03 浏览: 26
以下是使用Ceres实现牛顿法、高斯牛顿法和阻尼最小二乘法的示例代码:
牛顿法:
```c++
#include <iostream>
#include "ceres/ceres.h"
using namespace std;
// 定义残差函数
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(10.0) - x[0];
return true;
}
};
int main(int argc, char** argv) {
// 初始化问题
ceres::Problem problem;
// 添加变量
double x = 0.5;
problem.AddParameterBlock(&x, 1);
// 添加残差项
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor);
problem.AddResidualBlock(cost_function, nullptr, &x);
// 设置求解选项
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
options.minimizer_progress_to_stdout = true;
options.trust_region_strategy_type = ceres::LEVENBERG_MARQUARDT;
// 求解问题
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
// 输出结果
cout << summary.BriefReport() << endl;
cout << "x = " << x << endl;
return 0;
}
```
高斯牛顿法:
```c++
#include <iostream>
#include "ceres/ceres.h"
using namespace std;
// 定义残差函数
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(10.0) - x[0];
return true;
}
};
int main(int argc, char** argv) {
// 初始化问题
ceres::Problem problem;
// 添加变量
double x = 0.5;
problem.AddParameterBlock(&x, 1);
// 添加残差项
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor);
problem.AddResidualBlock(cost_function, nullptr, &x);
// 设置求解选项
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
options.minimizer_progress_to_stdout = true;
options.trust_region_strategy_type = ceres::DOGLEG;
// 求解问题
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
// 输出结果
cout << summary.BriefReport() << endl;
cout << "x = " << x << endl;
return 0;
}
```
阻尼最小二乘法:
```c++
#include <iostream>
#include "ceres/ceres.h"
using namespace std;
// 定义残差函数
struct CostFunctor {
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(10.0) - x[0];
return true;
}
};
int main(int argc, char** argv) {
// 初始化问题
ceres::Problem problem;
// 添加变量
double x = 0.5;
problem.AddParameterBlock(&x, 1);
// 添加残差项
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<CostFunctor, 1, 1>(new CostFunctor);
problem.AddResidualBlock(cost_function, nullptr, &x);
// 设置求解选项
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
options.minimizer_progress_to_stdout = true;
options.trust_region_strategy_type = ceres::DOGLEG;
options.dogleg_type = ceres::SUBSPACE_DOGLEG;
options.use_nonmonotonic_steps = true;
// 求解问题
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
// 输出结果
cout << summary.BriefReport() << endl;
cout << "x = " << x << endl;
return 0;
}
```
在这些示例中,我们将牛顿法、高斯牛顿法和阻尼最小二乘法的求解选项分别设置为LEVENBERG_MARQUARDT、DOGLEG和SUBSPACE_DOGLEG,并使用ceres::Solve函数求解问题。最后,我们输出求解结果。
相关推荐
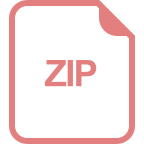
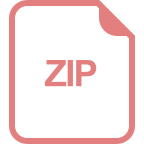
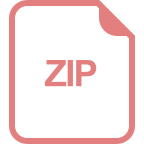














