ceres 实现阻尼最小二乘
时间: 2023-11-13 20:06:03 浏览: 157
在 Ceres Solver 中,实现阻尼最小二乘的方法有两种:
1. 通过在 `Problem` 中添加一个阻尼项来实现。假设我们的问题中有一组残差 $r_i$,那么阻尼最小二乘的目标函数可以表示为:
$$
\min \sum_{i=1}^n r_i^2 + \lambda \sum_{j=1}^m x_j^2
$$
其中 $\lambda$ 是阻尼因子,$x_j$ 是待优化的变量。可以通过在 `Problem` 中添加一个额外的残差项来实现上述目标函数的最小化。具体实现方式如下:
```c++
// 定义残差结构体
struct Residual {
Residual(double r, double lambda) : r_(r), lambda_(lambda) {}
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(r_);
for (int i = 0; i < 3; ++i) {
residual[0] += T(lambda_) * x[i] * x[i];
}
return true;
}
double r_;
double lambda_;
};
// 创建 Problem 对象,并添加残差项
ceres::Problem problem;
for (int i = 0; i < n; ++i) {
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<Residual, 1, 3>(new Residual(r[i], lambda));
problem.AddResidualBlock(cost_function, NULL, x);
}
// 配置 Solver 并求解
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
```
2. 通过在优化变量的参数块中添加一个阻尼项来实现。假设我们的问题中有一组残差 $r_i$,那么阻尼最小二乘的目标函数可以表示为:
$$
\min \sum_{i=1}^n r_i^2 + \lambda \sum_{j=1}^m x_j^2
$$
其中 $\lambda$ 是阻尼因子,$x_j$ 是待优化的变量。可以通过在优化变量的参数块中添加一个额外的变量来实现上述目标函数的最小化。具体实现方式如下:
```c++
// 定义残差结构体
struct Residual {
Residual(double r) : r_(r) {}
template <typename T>
bool operator()(const T* const x, T* residual) const {
residual[0] = T(r_);
return true;
}
double r_;
};
// 创建 Problem 对象,并添加残差项
ceres::Problem problem;
ceres::CostFunction* cost_function =
new ceres::AutoDiffCostFunction<Residual, 1, 4>(new Residual(r[i]));
problem.AddResidualBlock(cost_function, NULL, x, new double[1]{sqrt(lambda)});
// 配置 Solver 并求解
ceres::Solver::Options options;
options.linear_solver_type = ceres::DENSE_QR;
ceres::Solver::Summary summary;
ceres::Solve(options, &problem, &summary);
```
在上述代码中,我们将阻尼因子的平方根作为一个额外的变量添加到了优化变量的参数块中,然后在残差函数中使用该变量来计算阻尼项。
阅读全文
相关推荐
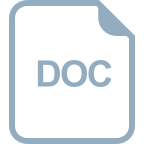
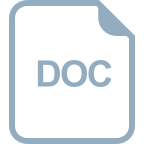
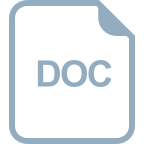








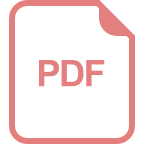
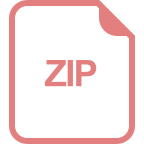
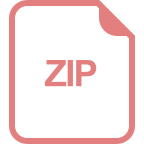



