python 中文语音翻译英文语音
时间: 2023-12-06 14:00:39 浏览: 46
在Python中,我们可以使用谷歌云端语音API来实现中文语音翻译为英文语音。以下是一个简单的实现过程:
首先,我们需要在谷歌云端API平台上创建一个项目,并启用谷歌云端语音转文字和谷歌云端文字转语音的API。
接下来,我们需要安装Python的谷歌云端语音库。可以使用pip命令进行安装:
```python
pip install google-cloud-speech
```
然后,我们还需要安装Python的文本翻译库。可以使用pip命令进行安装:
```python
pip install google-cloud-translate
```
接下来,我们需要编写Python代码。首先,我们使用谷歌云端语音库将中文语音转换为文本:
```python
from google.cloud import speech
def speech_to_text(audio_file):
client = speech.SpeechClient()
with open(audio_file, 'rb') as audio_file:
content = audio_file.read()
audio = speech.RecognitionAudio(content=content)
config = speech.RecognitionConfig(language_code='zh-CN')
response = client.recognize(config=config, audio=audio)
result = ''
for result in response.results:
result += result.alternatives[0].transcript
return result
```
然后,我们使用谷歌云端文本翻译库将中文文本翻译为英文:
```python
from google.cloud import translate
def translate_text(text):
client = translate.TranslationServiceClient()
target_language_code = 'en'
parent = f"projects/your-project-id/locations/global"
response = client.translate_text(
request={
"parent": parent,
"contents": [text],
"target_language_code": target_language_code,
}
)
result = response.translations[0].translated_text
return result
```
最后,我们可以将英文文本转换为英文语音并保存到本地:
```python
from google.cloud import texttospeech
import os
def text_to_speech(text, output_file):
client = texttospeech.TextToSpeechClient()
synthesis_input = texttospeech.SynthesisInput(text=text)
voice = texttospeech.VoiceSelectionParams(
language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.FEMALE
)
audio_config = texttospeech.AudioConfig(
audio_encoding=texttospeech.AudioEncoding.MP3
)
response = client.synthesize_speech(
request={
"input": synthesis_input,
"voice": voice,
"audio_config": audio_config,
}
)
with open(output_file, "wb") as out:
out.write(response.audio_content)
print(f'音频文件已保存为: {output_file}')
```
通过以上代码,我们可以实现将中文语音翻译为英文语音的功能。你只需将中文语音文件作为参数传递给`speech_to_text`函数,然后将返回的文本传递给`translate_text`函数,然后将得到的英文文本传递给`text_to_speech`函数即可。
需要注意的是,以上代码需要替换为你自己的谷歌云端API项目ID。你还需要准备一些中文语音文件进行测试,以及指定输出的英文语音文件路径。
相关推荐
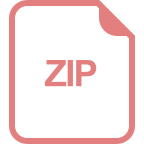
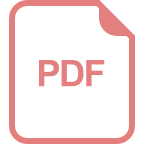
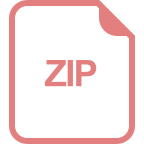














