c语言实现银行家算法的代码
时间: 2024-05-15 20:10:17 浏览: 136
银行家算法是一种避免死锁的算法,C语言实现银行家算法的代码如下:
```
#include <stdio.h>
#include <stdbool.h>
#define MAX_PROCESS 10
#define MAX_RESOURCE 10
int n, m;
int available[MAX_RESOURCE];
int maximum[MAX_PROCESS][MAX_RESOURCE];
int allocation[MAX_PROCESS][MAX_RESOURCE];
int need[MAX_PROCESS][MAX_RESOURCE];
bool finish[MAX_PROCESS];
bool is_safe() {
int work[MAX_RESOURCE];
for (int i = 0; i < m; i++) {
work[i] = available[i];
}
bool finish[MAX_PROCESS] = {false};
int safe_sequence[MAX_PROCESS], count = 0;
while (count < n) {
bool found = false;
for (int i = 0; i < n; i++) {
if (!finish[i]) {
bool can_allocate = true;
for (int j = 0; j < m; j++) {
if (need[i][j] > work[j]) {
can_allocate = false;
break;
}
}
if (can_allocate) {
finish[i] = true;
safe_sequence[count++] = i;
found = true;
for (int j = 0; j < m; j++) {
work[j] += allocation[i][j];
}
}
}
}
if (!found) {
return false;
}
}
printf("Safe sequence: ");
for (int i = 0; i < n; i++) {
printf("%d ", safe_sequence[i]);
}
printf("\n");
return true;
}
void request_resources(int process_id, int request[]) {
bool can_allocate = true;
for (int i = 0; i < m; i++) {
if (request[i] > need[process_id][i] || request[i] > available[i]) {
can_allocate = false;
break;
}
}
if (can_allocate) {
for (int i = 0; i < m; i++) {
available[i] -= request[i];
allocation[process_id][i] += request[i];
need[process_id][i] -= request[i];
}
if (is_safe()) {
printf("Request is satisfied.\n");
} else {
printf("Request is denied.\n");
for (int i = 0; i < m; i++) {
available[i] += request[i];
allocation[process_id][i] -= request[i];
need[process_id][i] += request[i];
}
}
} else {
printf("Request is denied.\n");
}
}
int main() {
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the number of resources: ");
scanf("%d", &m);
printf("Enter the number of available resources:\n");
for (int i = 0; i < m; i++) {
scanf("%d", &available[i]);
}
printf("Enter the maximum demand of each process:\n");
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
scanf("%d", &maximum[i][j]);
need[i][j] = maximum[i][j];
}
}
printf("Enter the allocated resources of each process:\n");
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
scanf("%d", &allocation[i][j]);
need[i][j] -= allocation[i][j];
}
}
is_safe();
int process_id;
printf("Enter process ID to request resources: ");
scanf("%d", &process_id);
int request[MAX_RESOURCE];
printf("Enter the requested resources:\n");
for (int i = 0; i < m; i++) {
scanf("%d", &request[i]);
}
request_resources(process_id, request);
return 0;
}
```
该代码实现了银行家算法的主要功能,包括判断系统是否处于安全状态以及处理进程请求资源。需要注意的是,此代码仅供参考,实际使用时需要根据具体情况进行修改和完善。
阅读全文
相关推荐
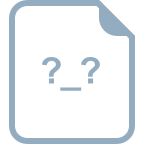
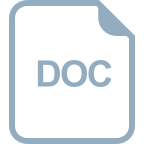
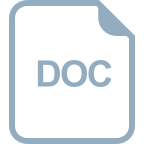
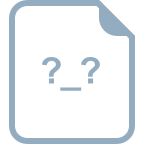
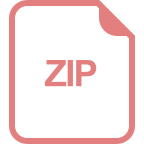
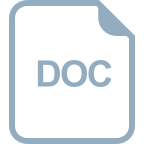
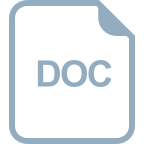









