spfa算法python
时间: 2023-11-09 12:03:38 浏览: 214
SPFA算法是一种用于解决单源最短路径问题的算法,它可以处理带有负权边的图。下面是SPFA算法的Python实现:
```python
from collections import deque
def spfa(graph, start):
dist = {node: float('inf') for node in graph}
dist[start] = 0
queue = deque([start])
while queue:
node = queue.popleft()
for neighbor in graph[node]:
new_dist = dist[node] + graph[node][neighbor]
if new_dist < dist[neighbor]:
dist[neighbor] = new_dist
if neighbor not in queue:
queue.append(neighbor)
return dist
```
其中,`graph`是一个字典,表示图的邻接表;`start`是起点。
相关问题
SPFA算法python
SPFA算法,全称是Shortest Path Faster Algorithm,即最短路径快速算法。它是一种单源最短路径算法,用于解决带权有向图中单个源点到其他所有点的最短路径问题。与Dijkstra算法相比,SPFA算法可以处理负权边,但是可能会出现负环的情况。
以下是SPFA算法的实现步骤:
1. 初始化:设置起点s的距离为0,其他点的距离为正无穷大;将起点s加入队列中。
2. 松弛操作:从队列中取出一个点u,对于u的所有出边(u, v),如果从起点s到v的距离可以通过从u到v的边缩短,则更新v的距离,并将v加入队列中。
3. 重复执行第2步,直到队列为空。
4. 若存在负环,则算法无法计算出最短路径;否则,最终得到起点s到各个点的最短路径长度。
以下是SPFA算法的Python实现:
```
def SPFA(graph, start):
queue = [start] # 初始化队列
dist = {node: float('inf') for node in graph} # 初始化距离
dist[start] = 0
while queue:
u = queue.pop(0)
for v in graph[u]:
if dist[v] > dist[u] + graph[u][v]:
dist[v] = dist[u] + graph[u][v]
if v not in queue:
queue.append(v)
return dist
```
其中graph是一个字典,表示有向图中每个点的出边和对应的权值。例如,`graph = {1: {2: 1, 3: 2}, 2: {3: 3, 4: 4}, 3: {4: -5}, 4: {}}`表示有向图中1号节点有两个出边分别指向2号节点和3号节点,权值分别为1和2。
SPFA算法python实现
SPFA算法是一种基于Bellman-Ford算法的单源最短路径算法,它可以处理负权边,但是会因为负环而陷入无限循环。下面是SPFA算法的Python实现:
```python
import collections
def spfa(graph, start):
"""
:param graph: 图的邻接表表示, graph[u]表示与顶点u相连的边和权重
:param start: 起点
:return: 从起点到其他顶点的最短距离
"""
# 初始化距离
distance = {vertex: float('inf') for vertex in graph}
distance[start] = 0
# 初始化队列
queue = collections.deque([start])
# 记录每个顶点的入队次数,防止出现负环
visit_count = {vertex: 0 for vertex in graph}
visit_count[start] = 1
# SPFA算法
while queue:
vertex = queue.popleft()
for neighbor, weight in graph[vertex]:
new_distance = distance[vertex] + weight
if new_distance < distance[neighbor]:
distance[neighbor] = new_distance
visit_count[neighbor] += 1
if visit_count[neighbor] > len(graph):
raise ValueError("图中存在负环")
queue.append(neighbor)
return distance
```
该算法使用了Python的collections模块中的deque实现队列,避免了使用list时的pop(0)操作的时间复杂度问题。同时,为了防止出现负环,记录了每个顶点的入队次数visit_count,当某个顶点的入队次数超过了图的顶点数,说明图中存在负环。
阅读全文
相关推荐



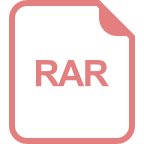











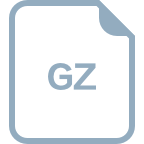