``` pointers to functions ```
时间: 2024-02-24 15:52:36 浏览: 102
这段代码是关于函数指针的介绍。函数指针是指向函数的指针变量,它可以用来存储和调用函数的地址。
在C语言中,函数名本身就是一个指向函数代码的指针。通过声明一个函数指针变量,可以将其指向特定的函数。例如:
```c
int add(int a, int b) {
return a + b;
}
int (*ptr)(int, int); // 声明一个函数指针变量
ptr = add; // 将函数指针变量指向add函数
int result = ptr(2, 3); // 通过函数指针调用add函数
```
在上面的代码中,我们首先定义了一个名为`add`的函数,它接受两个整数参数并返回它们的和。然后,我们声明了一个名为`ptr`的函数指针变量,它可以指向接受两个整数参数并返回整数的函数。接下来,我们将`ptr`指向`add`函数。最后,我们通过`ptr`调用`add`函数,并将结果存储在`result`变量中。
函数指针可以用于实现回调函数、动态选择要执行的函数等场景。它提供了一种灵活的方式来处理不同的函数逻辑。
相关问题
Write a program to prompt the user to enter a set of strings and the program would sort and display them in ascending alphabetical order. It is accepted to use the functions in the string.h library in the program. Here is a sample run. --------------------------------------------------------- How many strings you are going to enter?: 4 Enter Strings One by One: Nanjing Shanghai Chengdu Chongqing Order of Sorted Strings: Chengdu Chongqing Nanjing Shanghai ---------------------------------------------------------
Sure, here's a C program that prompts the user to enter a set of strings, sorts them in ascending alphabetical order, and displays them:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STR_LEN 100
#define MAX_STR_NUM 100
int compare_strings(const void* a, const void* b) {
return strcmp(*(const char**)a, *(const char**)b);
}
int main() {
int num_strings;
char buffer[MAX_STR_LEN];
char* strings[MAX_STR_NUM];
printf("How many strings you are going to enter?: ");
scanf("%d", &num_strings);
printf("Enter Strings One by One:\n");
for (int i = 0; i < num_strings; i++) {
scanf("%s", buffer);
strings[i] = strdup(buffer); // allocate memory and copy string
}
qsort(strings, num_strings, sizeof(char*), compare_strings);
printf("Order of Sorted Strings:\n");
for (int i = 0; i < num_strings; i++) {
printf("%s\n", strings[i]);
free(strings[i]); // free allocated memory
}
return 0;
}
```
The `compare_strings` function is used by `qsort` to compare two strings. The `strings` array is an array of pointers to strings, where each pointer points to a dynamically allocated string (using `strdup`). After sorting the strings, we simply print them out and free the memory allocated for each string.
The following is an attempt to create a class to represent information about pets: class Pet { public: Pet(); void printDescription(); string name; int type; bool neuterSpayed; bool talks; }; Pet::Pet() : type(0), neuterSpayed(false), talks(false) { } void Pet::printDescription() { switch (type) { case 0: cout << "Dog named " << name << endl; cout << "Neuter/Spayed: " << neuterSpayed; break; case 1: cout << "Cat named " << name << endl; cout << "Neuter/Spayed: " << neuterSpayed; break; case 2: cout << "Bird named " << name << endl; cout << "Talks: " << talks << endl; break; } cout << endl; } Rewrite this code using inheritance. You should have a Pet class with subclasses for Dog, Cat, and Bird. Variables should be associated with the appropriate classes, defined as private when appropriate, and have appropriate functions to access the variables. Rewrite the printDescription function as a virtual function. There should no longer be the need for a switch statement or a type variable. Write a main function that creates a vector or array of pets that includes at least one bird, one dog, and one cat, and then loops through and outputs a description of each one.
Here's the updated code:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Pet {
public:
Pet(string name) : m_name(name) {}
virtual void printDescription() = 0; // make it pure virtual to make Pet an abstract class
protected:
string m_name;
};
class Dog : public Pet {
public:
Dog(string name, bool neutered) : Pet(name), m_neutered(neutered) {}
void printDescription() override {
cout << "Dog named " << m_name << endl;
cout << "Neuter/Spayed: " << m_neutered << endl;
}
private:
bool m_neutered;
};
class Cat : public Pet {
public:
Cat(string name, bool neutered) : Pet(name), m_neutered(neutered) {}
void printDescription() override {
cout << "Cat named " << m_name << endl;
cout << "Neuter/Spayed: " << m_neutered << endl;
}
private:
bool m_neutered;
};
class Bird : public Pet {
public:
Bird(string name, bool talks) : Pet(name), m_talks(talks) {}
void printDescription() override {
cout << "Bird named " << m_name << endl;
cout << "Talks: " << m_talks << endl;
}
private:
bool m_talks;
};
int main() {
vector<Pet*> pets;
pets.push_back(new Dog("Fido", true));
pets.push_back(new Cat("Whiskers", false));
pets.push_back(new Bird("Polly", true));
for (Pet* pet : pets) {
pet->printDescription();
}
// free memory
for (Pet* pet : pets) {
delete pet;
}
return 0;
}
```
In this updated code, we've used inheritance to create subclasses for Dog, Cat, and Bird. Each subclass has its own private variables `m_neutered` and `m_talks`, respectively. The `printDescription` function is now a virtual function in the `Pet` class, which means that each subclass can override it with its own implementation.
In the main function, we create a vector of `Pet` pointers and add instances of each subclass to it. We then loop through the vector and call the `printDescription` function on each `Pet` object, which automatically calls the appropriate subclass implementation of the function. Finally, we free the memory allocated for each `Pet` object using `delete`.
阅读全文
相关推荐
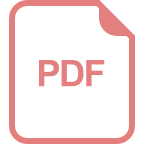
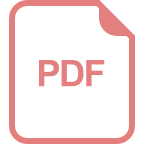
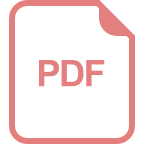
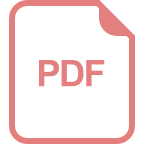
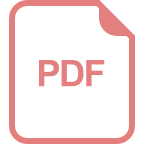
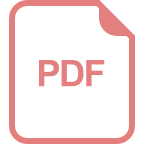
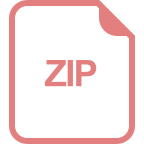
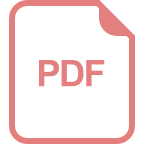
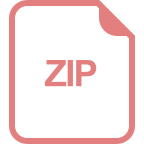
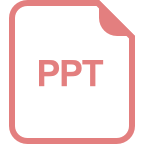
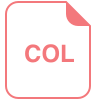
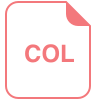
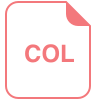
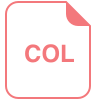
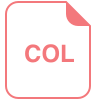

