python opencv blobFromImage
时间: 2024-03-23 07:34:45 浏览: 10
blobFromImage是OpenCV中的一个函数,用于将图像转换为神经网络所需的blob格式。它主要用于深度学习中的目标检测和图像分类任务。
该函数的语法如下:
```
cv2.dnn.blobFromImage(image, scalefactor=None, size=None, mean=None, swapRB=None, crop=None, ddepth=None)
```
参数说明:
- image:输入的图像。
- scalefactor:缩放因子,用于对图像进行缩放,默认为1.0。
- size:输出blob的尺寸,通常是神经网络模型的输入尺寸。
- mean:用于对图像进行均值减法的均值向量,一般是RGB三个通道的均值。
- swapRB:交换红色通道和蓝色通道,默认为False。
- crop:是否进行裁剪,默认为False。
- ddepth:输出blob的深度,默认为cv2.CV_32F。
使用blobFromImage函数可以将输入图像转换为神经网络所需的blob格式,方便进行后续的深度学习任务。在使用时,需要根据具体的模型和任务设置合适的参数。
相关问题
python opencv yolo
Python和OpenCV结合使用YOLO(You Only Look Once)可以实现目标检测和物体识别的功能。YOLO是一种基于深度学习的目标检测算法,它可以实时地在图像或视频中检测和定位多个对象。
要在Python中使用YOLO,首先需要安装OpenCV和YOLO的相关库。可以使用pip命令来安装它们:
```
pip install opencv-python
pip install opencv-contrib-python
```
接下来,需要下载YOLO的权重文件和配置文件。YOLO有几个不同版本,可以根据需求选择不同的模型。一般来说,比较常用的是YOLOv3或YOLOv4。
下载YOLOv3的权重文件和配置文件:
```
wget https://pjreddie.com/media/files/yolov3.weights
wget https://github.com/pjreddie/darknet/blob/master/cfg/yolov3.cfg
wget https://github.com/pjreddie/darknet/blob/master/data/coco.names
```
下载YOLOv4的权重文件和配置文件:
```
wget https://github.com/AlexeyAB/darknet/releases/download/darknet_yolo_v3_optimal/yolov4.weights
wget https://github.com/AlexeyAB/darknet/blob/master/cfg/yolov4.cfg
wget https://github.com/AlexeyAB/darknet/blob/master/data/coco.names
```
下载完毕后,可以使用下面的代码加载模型并进行目标检测:
```python
import cv2
# 加载模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# 加载类别标签
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 加载图像
image = cv2.imread("image.jpg")
# 进行目标检测
blob = cv2.dnn.blobFromImage(image, 1/255, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
outs = net.forward(net.getUnconnectedOutLayersNames())
# 解析检测结果
for out in outs:
for detection in out:
scores = detection[5:]
classId = np.argmax(scores)
confidence = scores[classId]
if confidence > 0.5:
centerX = int(detection[0] * width)
centerY = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(centerX - w / 2)
y = int(centerY - h / 2)
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(image, classes[classId], (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Image", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码会加载YOLO模型和类别标签,然后读取一张图像,进行目标检测,并在图像中绘制检测结果的边界框和类别标签。最后,显示图像并等待按键退出。
请注意,上述代码只提供了一个基本的示例,实际应用中可能需要根据具体需求进行更多的调整和优化。
yolov3 python opencv
YoloV3 is an object detection algorithm that can be implemented using Python and OpenCV. Here are the steps to implement YoloV3 using Python and OpenCV:
1. Download the YoloV3 weights and configuration files from the official website.
2. Install OpenCV and other necessary libraries.
3. Load the YoloV3 network using OpenCV's DNN module.
4. Read the input image or video frame.
5. Preprocess the input image by resizing it to the required size and normalizing the pixel values.
6. Pass the preprocessed image through the YoloV3 network to obtain the predicted bounding boxes, class labels, and confidence scores.
7. Filter the predicted bounding boxes based on their confidence scores and non-maximum suppression.
8. Draw the filtered bounding boxes and class labels on the input image.
9. Display the output image or save it to a file.
Here's a sample code snippet for implementing YoloV3 using Python and OpenCV:
```
import cv2
# Load YoloV3 network
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# Load classes
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# Set input and output layers
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# Read input image
img = cv2.imread("input.jpg")
# Preprocess image
blob = cv2.dnn.blobFromImage(img, scalefactor=0.00392, size=(416,416), mean=(0,0,0), swapRB=True, crop=False)
# Pass image through YoloV3 network
net.setInput(blob)
outs = net.forward(output_layers)
# Get predicted bounding boxes, class labels, and confidence scores
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * img.shape[1])
center_y = int(detection[1] * img.shape[0])
w = int(detection[2] * img.shape[1])
h = int(detection[3] * img.shape[0])
x = center_x - w // 2
y = center_y - h // 2
class_ids.append(class_id)
confidences.append(float(confidence))
boxes.append([x, y, w, h])
# Apply non-maximum suppression
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# Draw filtered bounding boxes and class labels on input image
for i in indices:
i = i[0]
x, y, w, h = boxes[i]
label = classes[class_ids[i]]
cv2.rectangle(img, (x, y), (x + w, y + h), (0,255,0), 2)
cv2.putText(img, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0,255,0), 2)
# Display output image
cv2.imshow("Output", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
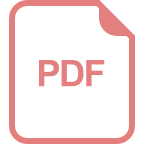












