python opencv 物体检测算法获得目标框和类别信息 并存储到list中
时间: 2024-02-23 10:02:18 浏览: 78
好的,可以使用OpenCV中的物体检测算法来实现。常用的算法有Haar分类器、HOG+SVM、YOLO等。
以YOLO为例,可以使用以下步骤来获得目标框和类别信息并存储到list中:
1. 加载YOLO模型。可以使用已经训练好的模型,也可以自己训练。
2. 读取待检测的图像,并将其转换为模型需要的格式。
3. 对图像进行物体检测,获得检测结果(目标框和类别信息)。
4. 遍历检测结果,将目标框和类别信息存储到list中。
下面是示例代码:
```python
import cv2
# 加载YOLO模型
net = cv2.dnn.readNetFromDarknet("yolov3.cfg", "yolov3.weights")
# 读取待检测的图像
img = cv2.imread("test.jpg")
# 将图像转换为模型需要的格式
blob = cv2.dnn.blobFromImage(img, 1/255.0, (416, 416), swapRB=True, crop=False)
# 对图像进行物体检测
net.setInput(blob)
outputs = net.forward(net.getUnconnectedOutLayersNames())
# 遍历检测结果,将目标框和类别信息存储到list中
results = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * img.shape[1])
center_y = int(detection[1] * img.shape[0])
width = int(detection[2] * img.shape[1])
height = int(detection[3] * img.shape[0])
left = int(center_x - width / 2)
top = int(center_y - height / 2)
results.append([left, top, width, height, class_id])
# 打印结果
print(results)
```
需要注意的是,上述代码中使用的是yolov3模型,需要先下载对应的配置文件(yolov3.cfg)和权重文件(yolov3.weights)。同时,上述代码中只保留了置信度大于0.5的检测结果,可以根据需要进行调整。
阅读全文
相关推荐
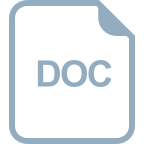
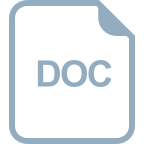
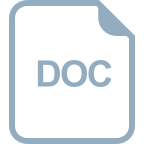




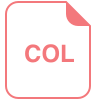
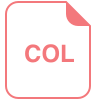




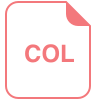




