python读取文件,找到文件中最长的字符串,并插入文件的最后
时间: 2024-10-17 17:12:50 浏览: 18
在Python中,你可以通过以下几个步骤来实现这个功能:
1. 打开文件并读取内容:首先需要打开文件,可以使用内置的`open()`函数。如果文件很大,可以考虑使用逐行读取的方式,因为一次性加载整个文件可能会消耗大量内存。
```python
with open('filename.txt', 'r') as file:
lines = file.readlines()
```
2. 找到最长的字符串:遍历每一行,计算每个字符串的长度,并记录下当前最长的字符串及其长度。
```python
max_length = 0
longest_string = ''
for line in lines:
if len(line) > max_length:
max_length = len(line)
longest_string = line.strip() # 移除行尾的换行符
```
3. 插入最长的字符串到文件末尾:找到最长字符串后,关闭原来的文件,然后以追加模式`'a'`打开,将最长字符串添加到文件的末尾。
```python
with open('filename.txt', 'a') as file:
file.write(longest_string + '\n')
```
完整的代码示例:
```python
# 假设已有一个变量lines存储了文件内容
if lines:
max_length = 0
for line in lines:
length = len(line.strip())
if length > max_length:
max_length = length
longest_string = line
with open('filename.txt', 'a') as file:
file.write(longest_string + '\n')
else:
print("文件为空或未读取")
```
阅读全文
相关推荐
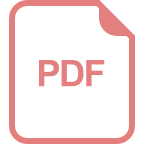
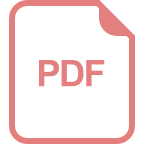
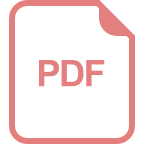
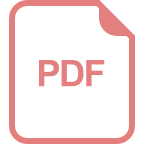
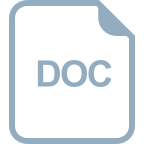
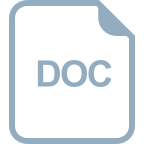
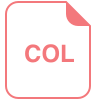
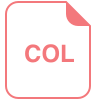
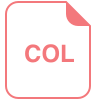
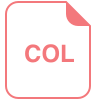
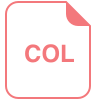
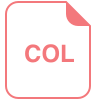
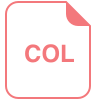





