用c语言或者c++实现哈希表的插入、删除和查找操作
时间: 2024-10-13 07:13:20 浏览: 3
在C/C++中实现哈希表(也称为散列表),通常涉及以下几个步骤:
1. 插入操作:
- 首先,你需要创建一个大小固定并预先填充哈希函数的数组,这个函数会将输入的数据映射到数组的一个索引上。
- 当插入数据时,计算给定键值对的哈希码,并取模数组长度,得到存储位置(解决哈希冲突的一种常见方法是使用开放寻址法或链地址法)。
- 如果对应位置是空的,直接将元素插入;如果已存在元素,就需要处理冲突。例如,在链地址法下,可以在该位置添加一个新的节点。
```cpp
struct HashNode {
key_type key;
value_type value;
HashNode* next;
};
class HashTable {
private:
size_t size;
HashNode** table;
public:
void insert(const key_type& key, const value_type& value) {
size_t index = hash_function(key) % size; // 哈希函数
if (table[index] == nullptr) {
table[index] = new HashNode{key, value, nullptr};
} else {
HashNode* current = table[index];
while (current->next != nullptr) {
current = current->next;
}
current->next = new HashNode{key, value, nullptr}; // 如果有冲突,追加到链表末尾
}
}
}
```
2. 删除操作:
- 使用相同的哈希函数找到待删除元素的位置。
- 检查当前位置是否为空,如果不为空,对比当前元素的键是否匹配。
- 匹配则从链表中移除该元素;若链表为空,则需要清理节点。
```cpp
void remove(const key_type& key) {
size_t index = hash_function(key) % size;
if (table[index]) {
HashNode* current = table[index];
HashNode* previous = nullptr;
while (current != nullptr && current->key != key) {
previous = current;
current = current->next;
}
if (current != nullptr) {
if (previous == nullptr) {
table[index] = current->next;
} else {
previous->next = current->next;
}
delete current;
}
}
}
```
3. 查找操作:
- 根据键计算哈希索引,然后遍历对应位置的链表,直到找到匹配项或遍历完链表。
```cpp
value_type find(const key_type& key) {
size_t index = hash_function(key) % size;
HashNode* current = table[index];
while (current != nullptr) {
if (current->key == key) {
return current->value;
}
current = current->next;
}
return value_type(); // 或者抛异常表示未找到
}
```
相关推荐
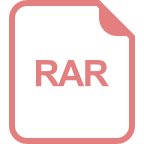
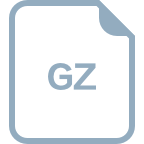
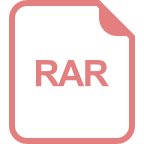
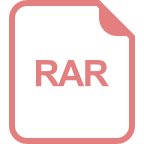
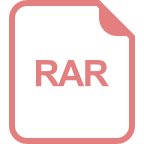



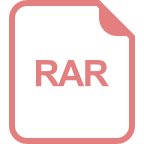
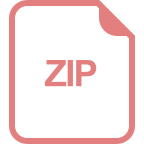
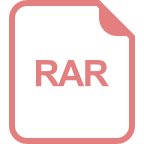
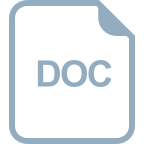
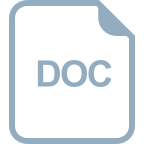



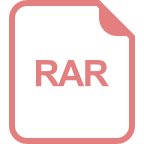
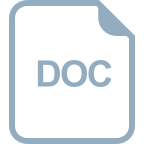
