java8的ConcurrentHashMap的put方法过程
时间: 2023-08-13 12:08:10 浏览: 49
Java 8 的 ConcurrentHashMap 类是线程安全的哈希表实现,它的 put 方法用于将键值对添加到哈希表中。下面是 ConcurrentHashMap 的 put 方法的大致过程:
1. 首先,根据给定的键计算出哈希值。
2. 根据哈希值确定键值对应的段(Segment)。
3. 在段中加锁,以确保线程安全性。
4. 检查段中是否已经存在该键。如果存在,则更新对应的值,并返回旧值。
5. 如果不存在该键,则创建一个新的节点,并将其插入到段中。
6. 如果插入操作导致段中的节点数量超过阈值(默认为 8),则进行扩容操作,将该段中的节点重新分配到新的段中。
7. 最后释放段的锁,并返回旧值(如果存在)。
需要注意的是,ConcurrentHashMap 通过将整个哈希表分割成多个段来实现并发访问的效果。每个段都拥有自己的锁,不同的线程可以同时访问不同的段,从而提高并发性能。这也意味着在多线程环境下,不同的线程可以同时执行 put 操作,而不会相互阻塞。
以上是 Java 8 的 ConcurrentHashMap 的 put 方法的简要过程描述。具体实现细节可能因版本和具体实现而有所不同。
相关问题
ConcurrentHashMap put 方法过程
ConcurrentHashMap是线程安全的哈希表,put方法是ConcurrentHashMap中最重要的方法之一。下面是ConcurrentHashMap put方法的过程:
1. 首先,ConcurrentHashMap会根据key的hash值和table数组的长度计算出key在table数组中的位置,如果该位置为空,则直接在该位置插入新的键值对。
2. 如果该位置已经存在键值对,则需要进行以下操作:
a. 如果该位置的节点是链表,则遍历链表,找到key相同的节点,将其value更新为新的值。
b. 如果该位置的节点是红黑树,则在红黑树中查找key相同的节点,将其value更新为新的值。
c. 如果该位置的节点既不是链表也不是红黑树,则说明该位置的节点是一个单独的节点,需要将该节点转化为链表或红黑树。
3. 如果插入新的键值对后,ConcurrentHashMap中的键值对数量超过了负载因子(load factor)所允许的最大值,则需要进行扩容操作。
4. 在插入新的键值对时,ConcurrentHashMap使用了一种叫做“分段锁”的技术,将整个哈希表分成了多个段(segment),每个段都有自己的锁。这样,在插入新的键值对时,只需要锁住对应的段,而不需要锁住整个哈希表,从而提高了并发性能。
下面是一个示例代码:
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("key1", 1);
map.put("key2", 2);
```
concurrenthashmap的put过程
### 回答1:
ConcurrentHashMap的put操作过程如下:
1. 首先,ConcurrentHashMap会根据键的哈希值定位到对应的Segment。Segment是ConcurrentHashMap内部的一个数据结构,它类似于HashMap的一个子集,是一个独立的哈希表。
2. 在找到对应的Segment后,ConcurrentHashMap会加锁(ReentrantLock)来保证线程安全。加锁操作的粒度是Segment级别的,即同一时刻只有一个线程能够访问该Segment内部的数据结构。
3. 接着,ConcurrentHashMap会在该Segment中查找指定的键。如果找到了对应的键,那么它会用新值替换旧值,并返回旧值。
4. 如果在该Segment中没有找到指定的键,那么ConcurrentHashMap会创建一个新的Entry(键值对),并将其插入到Segment中。如果Segment中已经有太多的元素,那么就会进行扩容操作。
5. 最后,ConcurrentHashMap会释放锁,允许其他线程访问该Segment。
需要注意的是,ConcurrentHashMap的put操作是线程安全的,即多个线程可以同时进行put操作而不会出现数据冲突。这是因为ConcurrentHashMap将内部的数据结构分成了多个Segment,并对每个Segment进行了加锁。这样就可以在多个线程之间实现细粒度的并发控制,从而提高了ConcurrentHashMap的并发性能。
### 回答2:
ConcurrentHashMap是Java中线程安全的哈希表实现,相比于HashMap,它允许多个线程同时操作哈希表,能够提高并发性能,但同时也会增加一些开销。在put操作时,ConcurrentHashMap需要做以下几个步骤:
1.计算键的哈希值。
put操作需要先根据键的哈希值来确定它在哈希表中的位置。ConcurrentHashMap使用类似于HashMap的桶结构存储键值对,每个桶里有一个链表或红黑树来保存相同哈希值的键值对。
2.选择桶并加锁。
根据步骤1计算出的哈希值,选择要插入的桶。由于多线程同时操作,ConcurrentHashMap需要对选中的桶加锁,确保当前线程能够独占这个桶,避免出现并发问题。
3.在桶中查找并更新键值对。
在选中的桶中,通过遍历链表或红黑树来查找键值对。如果找到了原有的键值对,就更新它的值。如果没有找到,则创建一个新的键值对并插入到链表或红黑树中。
4.释放锁。
在完成插入操作后,需要将选中的桶解锁,让其他线程有机会操作这个桶。这里有必要提到ConcurrentHashMap使用分段锁机制,不同的桶可以独立加锁和释放锁,避免了整个哈希表被锁死的情况。
总的来说,ConcurrentHashMap的put操作涉及到的步骤比HashMap要多,但是可以保证多个线程并发操作时的线程安全,适用于高并发的多线程环境。同时,ConcurrentHashMap也支持读写分离,读操作不需要加锁,可以提高读操作的性能。
### 回答3:
ConcurrentHashMap是线程安全的HashMap实现,其put方法是保证线程安全的关键。该方法采用了锁分段技术来实现高效并发操作。下面是ConcurrentHashMap的put过程:
1. 根据key的哈希值获取其对应的Segment(锁段)。
2. 如果Segment为空则新建一个,如果已存在则直接进入下一步。
3. 在Segment中加锁,保证线程安全。
4. 判断该key是否已经存在,如果已存在则直接替换其对应的value值。
5. 如果key不存在,则在Segment中新建一个节点,并将key-value插入节点中。
6. 判断Segment中元素数量是否已经达到临界值,如果是则触发扩容操作。
7. 释放锁。
需要注意的是,Segment是ConcurrentHashMap中的核心数据结构之一,它将哈希表分成了多个锁段,每个锁段都是一个独立的HashMap。每个Segment都维护着自己的元素数量和扩容状态,这样就可以避免了全表加锁带来的性能瓶颈。
ConcurrentHashMap的put方法利用了锁分段技术,保证了线程安全且性能优秀。在常见的并发场景下,ConcurrentHashMap可以避免频繁的锁竞争,大大提高了程序的并发性能。
相关推荐
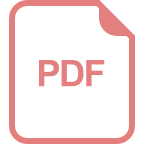
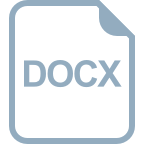
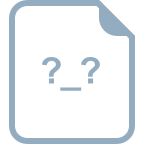
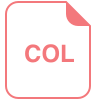
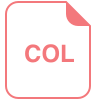










