完成以下头指针为L的单链表的设计与实现,要求完成单链表的初始化、头插或尾插法的创建、单链表所有数据元素显示与输出、并且在单链表查找值'F'的数据是否存在。同时编写主函数运行实现相应功能。用C语言写
时间: 2024-10-18 18:03:58 浏览: 22
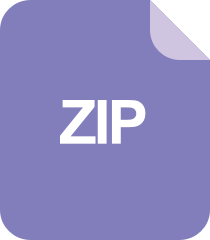
c++头插法和尾插法创建、删除、遍历单链表.zip
在C语言中,我们可以设计一个简单的单链表结构以及相关的操作。首先,我们需要定义一个节点结构体,包含数据域和指向下一个节点的指针。然后,我们将实现以下功能:
1. **节点结构体定义**:
```c
typedef struct ListNode {
int data; // 数据域
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
```
2. **初始化链表**:
```c
ListNode* createList() {
ListNode* head = NULL; // 初始化链表为空
return head;
}
```
3. **头插法或尾插法创建节点**:
- **头插法插入新节点**:
```c
void insertAtHead(ListNode** head, int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
```
- **尾插法插入新节点**:
```c
void insertAtTail(ListNode** head, int value) {
if (*head == NULL) { // 如果链表为空,直接插入头节点
insertAtHead(head, value);
return;
}
ListNode* tail = *head;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = (ListNode*)malloc(sizeof(ListNode)); // 插入新的节点
tail->next->data = value;
tail->next->next = NULL;
}
```
4. **遍历并输出链表**:
```c
void printList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
```
5. **查找值'F'的数据是否存在**:
```c
int searchForValue(ListNode* head, int value) {
ListNode* current = head;
while (current != NULL) {
if (current->data == value)
return 1; // 找到返回1
current = current->next;
}
return 0; // 没找到返回0
}
```
**主函数**:
```c
int main() {
ListNode* list = createList();
// 插入节点示例
insertAtHead(&list, 1); // [1]
insertAtTail(&list, 2); // [1] -> [2]
insertAtHead(&list, 3); // [3] -> [1] -> [2]
// 输出链表
printList(list);
// 查找 'F'
int found = searchForValue(list, 4); // 假设 'F' 不存在于链表中
printf("寻找值 'F' 是否存在: %d\n", found);
// 清理内存
while (list != NULL) {
ListNode* temp = list;
list = list->next;
free(temp);
}
return 0;
}
```
在这个例子中,我们完成了单链表的基本操作,并在主函数中展示了如何使用它们。注意,在实际使用中,记得处理内存分配失败的情况,并在结束时释放不再需要的内存。
阅读全文
相关推荐
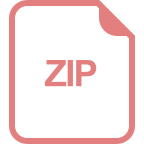
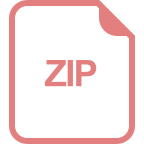















