c语言 要求实现一个函数,根据输入的字符串用尾插法创建带头结点的单链表
时间: 2024-10-24 20:02:27 浏览: 10
在C语言中,你可以通过以下几个步骤实现一个函数来使用尾插法创建带头结点的单链表。这里假设链表节点结构体定义为`typedef struct ListNode`:
```c
typedef struct ListNode {
char data; // 节点数据
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
```
函数名为`createListByTailInsertion`,它接受一个指向字符数组`char str[]`的指针作为输入,然后遍历字符串并添加每个字符到链表的尾部:
```c
ListNode* createListByTailInsertion(char str[]) {
// 创建头结点
ListNode* head = (ListNode*)malloc(sizeof(ListNode)); // 分配内存
head->data = '\0'; // 初始化头结点的数据为空(通常用于表示空列表)
head->next = NULL;
// 遍历输入字符串
for(int i = 0; str[i] != '\0'; ++i) { // 去除最后一个'\0',因为它是字符串结束标志
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = str[i]; // 将字符存入新节点
newNode->next = NULL; // 新节点默认无后续节点
// 尾插入操作
if(head->next == NULL) {
head->next = newNode;
} else {
ListNode* current = head->next;
while(current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
return head;
}
```
阅读全文
相关推荐
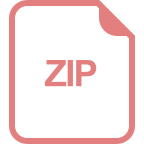
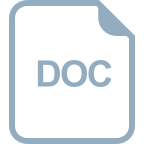
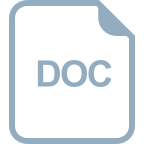
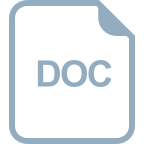















