c++中数组排序函数
时间: 2024-06-13 07:09:44 浏览: 25
C++中有多种数组排序函数,以下是两种常用的排序方法:
1. 冒泡排序
冒泡排序是一种简单的排序算法,它重复地遍历要排序的数列,一次比较两个元素,如果它们的顺序错误就把它们交换过来。遍历数列的工作是重复地进行直到没有再需要交换的元素。
示例代码:
```c++
#include <iostream>
using namespace std;
int main() {
int arr[9] = { 4,2,8,0,5,7,1,3,9 };
for (int i = 0; i < 9 - 1; i++) {
for (int j = 0; j < 9 - 1 - i; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
for (int i = 0; i < 9; i++) {
cout << arr[i] << endl;
}
return 0;
}
```
2. 快速排序
快速排序是一种常用的排序算法,采用了分治的思想,将原始数组分成较小和较大的两个子数组,然后递归地排序两个子数组。
示例代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[9] = { 4,2,8,0,5,7,1,3,9 };
sort(arr, arr + 9);
for (int i = 0; i < 9; i++) {
cout << arr[i] << endl;
}
return 0;
}
```
相关推荐
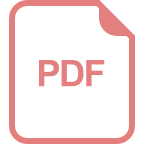
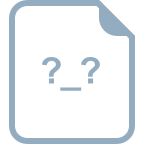
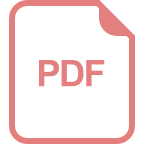
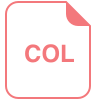
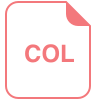
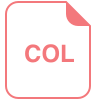
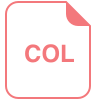
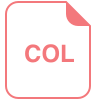









