c++实现利用二叉树的链式存储结构计算二叉树的深度完整代码
时间: 2024-11-06 11:10:01 浏览: 30
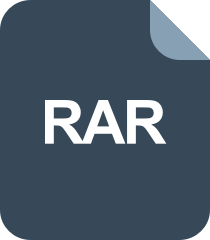
二叉树的链式存储结构-二叉链表

在C++中,我们可以使用递归的方式来实现二叉树的链式存储结构(即每个节点包含左指针、右指针以及指向下一个节点的指针)的深度计算。这里是一个简单的示例:
```cpp
#include <iostream>
// 定义二叉树节点的结构体
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode* next; // 链接其他节点的指针
};
// 用于计算树的深度
int maxDepth(TreeNode* root) {
if (root == nullptr) { // 如果根节点为空,则树的深度为0
return 0;
}
// 递归计算左子树和右子树的深度,并取较大值
int left_depth = maxDepth(root->left);
int right_depth = maxDepth(root->right);
// 树的深度等于左右子树深度的最大值加1(当前节点)
return std::max(left_depth, right_depth) + 1;
}
int main() {
// 创建一个示例二叉树
TreeNode* root = new TreeNode();
root->val = 1;
root->left = new TreeNode();
root->left->val = 2;
root->left->next = new TreeNode();
root->left->next->val = 3;
root->left->next->next = nullptr;
// 初始化右子树
root->right = new TreeNode();
root->right->val = 4;
root->right->next = new TreeNode();
root->right->next->val = 5;
root->right->next->next = nullptr;
// 计算并输出树的深度
int depth = maxDepth(root);
std::cout << "The maximum depth of the binary tree is: " << depth << std::endl;
// 清理内存
delete root;
return 0;
}
```
在这个例子中,`maxDepth` 函数会遍历整棵树并返回最大深度。
阅读全文
相关推荐
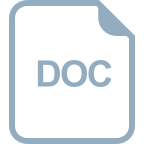
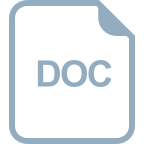



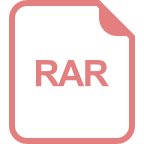
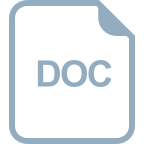
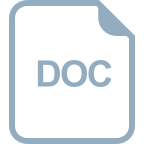
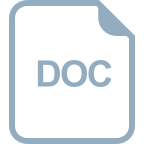
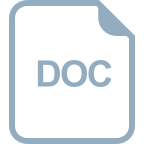
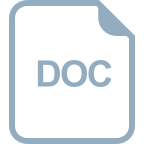





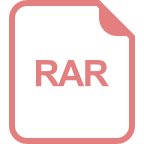
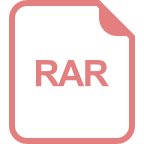