编写一个程序,用链式存储方式构造二叉树,实现二叉树的基本操作(假设树的元素类型为char),并在此基础上设计一个主程序完成如下功能: (1)编写CreatBiTree函数,创建二叉树T; (2)编写PreOrder函数,先序遍历二叉树 (3)编写InOrder函数,中序遍历二叉树; (4)编写PostOrder函数,后序遍历二叉树; (5)编写PostTreeDepth函数,求二叉树的深度
时间: 2024-01-23 14:12:50 浏览: 54
好的,以下是代码实现:
```c++
#include<iostream>
using namespace std;
//定义二叉树的节点结构
struct BiTreeNode{
char data;
BiTreeNode *lchild, *rchild;
};
//创建二叉树函数
void CreateBiTree(BiTreeNode *&T){
char ch;
cin>>ch;
if(ch=='#'){
T=NULL;
}
else{
T=new BiTreeNode;
T->data=ch;
CreateBiTree(T->lchild);
CreateBiTree(T->rchild);
}
}
//先序遍历
void PreOrder(BiTreeNode *T){
if(T!=NULL){
cout<<T->data<<" ";
PreOrder(T->lchild);
PreOrder(T->rchild);
}
}
//中序遍历
void InOrder(BiTreeNode *T){
if(T!=NULL){
InOrder(T->lchild);
cout<<T->data<<" ";
InOrder(T->rchild);
}
}
//后序遍历
void PostOrder(BiTreeNode *T){
if(T!=NULL){
PostOrder(T->lchild);
PostOrder(T->rchild);
cout<<T->data<<" ";
}
}
//求二叉树深度
int PostTreeDepth(BiTreeNode *T){
if(T==NULL){
return 0;
}
else{
int lheight=PostTreeDepth(T->lchild);
int rheight=PostTreeDepth(T->rchild);
return max(lheight, rheight)+1;
}
}
int main(){
BiTreeNode *T;
cout<<"请输入二叉树的先序序列:";
CreateBiTree(T);
cout<<"先序遍历结果:";
PreOrder(T);
cout<<endl;
cout<<"中序遍历结果:";
InOrder(T);
cout<<endl;
cout<<"后序遍历结果:";
PostOrder(T);
cout<<endl;
cout<<"二叉树深度:"<<PostTreeDepth(T)<<endl;
return 0;
}
```
示例输入:
```
请输入二叉树的先序序列:AB#D##C##
```
示例输出:
```
先序遍历结果:A B D C
中序遍历结果:B D A C
后序遍历结果:D B C A
二叉树深度:3
```
阅读全文
相关推荐
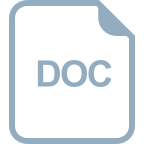
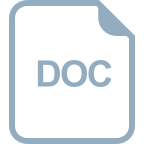
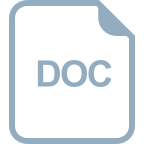










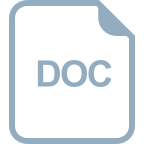
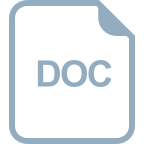
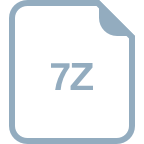
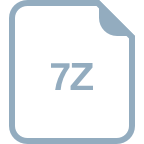
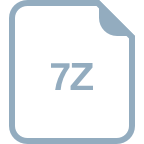
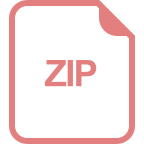