2. 定义一个函数,在上述二叉树中实现查找,若查找的元素在二叉树中存在,则得出该元素所在的层数,若不存在,则得出0。例如:在上述链式存储实现的二叉树中,查找元素, (1)若查找元素“H”,则得出所在层数4; (2)若查找元素“S”,则得出0,表示该元素在树中不存在。
时间: 2024-12-23 14:27:40 浏览: 2
在链式存储的二叉树中实现查找并计算元素所在层数,你需要遍历二叉树并在遍历过程中记录节点深度。对于每个节点,如果找到了目标元素,就递增层数并继续向下搜索左子树和右子树;如果没有找到,返回当前层数。这里的关键在于正确地维护深度信息。
以下是一个简单的C语言函数,假设我们有一个`Node`结构体,其中包含`value`, `left`, 和 `right` 子节点指针:
```c
#include <stdio.h>
#include <stdlib.h>
// 假设 Node 结构如下:
typedef struct Node {
char value;
struct Node* left;
struct Node* right;
} Node;
int findAndCountDepth(Node* root, char target, int depth) {
if (root == NULL) return depth; // 如果到达空节点,结束并返回当前层数
// 检查当前节点是否为目标元素
if (root->value == target) {
printf("Element found at depth %d\n", depth); // 打印结果并返回层数
return depth;
}
// 否则递归遍历左右子树
int left_depth = findAndCountDepth(root->left, target, depth + 1);
int right_depth = findAndCountDepth(root->right, target, depth + 1);
// 返回两子树中最深的那个节点的层数
return left_depth > right_depth ? left_depth : right_depth;
}
// 示例用法:
Node* buildTree(); // 假设这是构建二叉树的函数
char target;
int main() {
Node* root = buildTree();
target = 'H'; // 或者其他你要查找的目标字符
int result = findAndCountDepth(root, target, 0);
// 根据result判断元素是否存在以及层数
if (result == 0) {
printf("Element not found.\n");
} else {
// do something with the depth
}
free(root); // 不要忘记释放内存
return 0;
}
```
这个函数首先检查根节点,然后递归地对左右子树进行查找。如果找到目标元素,立即返回其层数;如果没有找到,则返回子树中的更深一层。最后在主函数中调用这个函数并处理返回的结果。
阅读全文
相关推荐
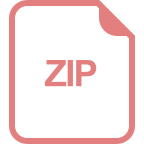
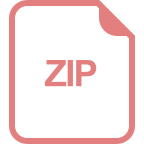
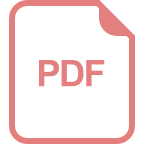

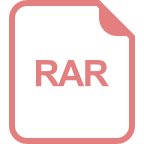
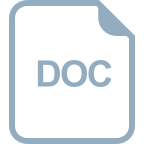
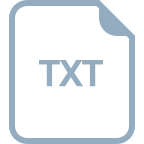
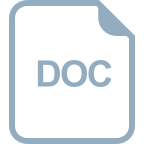
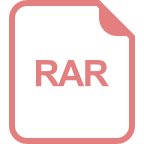
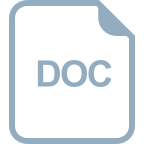
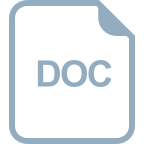
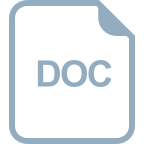
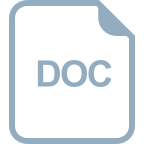
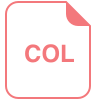
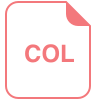
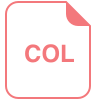
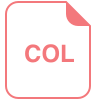

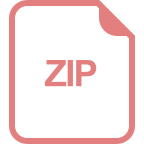