peewee 多条件查询
时间: 2023-09-04 09:14:25 浏览: 354
在Peewee中,可以使用多个条件来进行查询。以下是几种常见的多条件查询的示例:
1. 使用多个`where()`方法:
```python
from peewee import *
# 创建数据库连接
db = SqliteDatabase('my_database.db')
# 定义模型类
class Person(Model):
name = CharField()
age = IntegerField()
city = CharField()
class Meta:
database = db
# 查询名字为Alice,年龄为25的人
persons = Person.select().where((Person.name == 'Alice') & (Person.age == 25))
for person in persons:
print(person.name, person.age, person.city)
```
2. 使用`and_()`和`or_()`方法:
```python
from peewee import *
from peewee import fn
# 创建数据库连接
db = SqliteDatabase('my_database.db')
# 定义模型类
class Person(Model):
name = CharField()
age = IntegerField()
city = CharField()
class Meta:
database = db
# 查询名字为Alice并且年龄小于30的人,者名字为Bob的人
persons = Person.select().where(((Person.name == 'Alice') & (Person.age < 30)) | (Person.name == 'Bob'))
for person in persons:
print(person.name, person.age, person.city)
```
3. 使用`filter()`方法:
```python
from peewee import *
# 创建数据库连接
db = SqliteDatabase('my_database.db')
# 定义模型类
class Person(Model):
name = CharField()
age = IntegerField()
city = CharField()
class Meta:
database = db
# 查询年龄在25到30之间,并且城市为New York的人
persons = Person.select().filter((Person.age >= 25) & (Person.age <= 30) & (Person.city == 'New York'))
for person in persons:
print(person.name, person.age, person.city)
```
在这些示例中,我们创建了一个`Person`模型类来表示人的信息。然后使用不同的条件来查询数据库中符合条件的记录。
请根据你的具体需求,选择适合的查询方式来构建多条件查询。
阅读全文
相关推荐
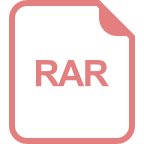


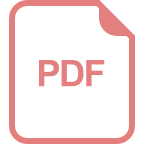
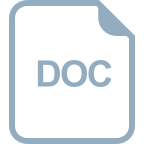
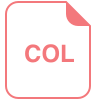
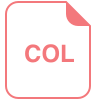
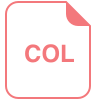
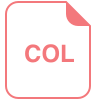







