本题要求实现带头结点的循环单链表的创建和单链表的区间删除。L是一个带头结点的循环单链表,函数ListCreate_CL用于创建一个循环单链表,函数ListDelete_CL用于删除取值大于min小于max的链表元素。完整代码
时间: 2024-10-14 18:11:08 浏览: 12
在C语言中,为了实现带头结点的循环单链表(Circular Linked List,CLL)以及相关的创建(ListCreate_CL)和区间删除(ListDelete_CL)操作,你需要首先定义链表节点结构、循环链表的头指针,并实现两个函数。
1. **链表节点定义**:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. **循环链表结构体**:
```c
typedef struct CLL {
Node* head;
Node* tail; // 指向最后一个节点的指针
} CircularLinkedList;
```
3. **创建循环单链表函数(ListCreate_CL)**:
```c
CircularLinkedList* ListCreate_CL() {
CircularLinkedList* list = (CircularLinkedList*)malloc(sizeof(CircularLinkedList));
list->head = list->tail = (Node*)malloc(sizeof(Node)); // 初始化头尾节点
list->head->next = list->head; // 创建循环链接
list->head->data = 0; // 首节点数据可自定义
return list;
}
```
4. **区间删除函数(ListDelete_CL)**:
```c
void ListDelete_CL(CircularLinkedList* list, int min, int max) {
if (!list || !list->head) return; // 空链表处理
Node* current = list->head;
// 循环遍历直到找到满足条件的节点或超出范围
while (current->next != list->head && current->data < min || current->data > max) {
current = current->next;
}
if (current->data >= min && current->data <= max) { // 节点在范围内
// 如果要删除的是头节点,更新头和尾指针
if (current == list->head) {
if (current == list->tail) {
list->head = NULL;
list->tail = NULL;
} else {
list->head = current->next;
list->tail->next = list->head;
}
} else { // 删除普通节点
current->prev->next = current->next;
if (current == list->tail) {
list->tail = current->prev;
}
}
free(current); // 释放内存
}
}
```
注意:以上代码假设`current->prev`是指当前节点前一个节点的指针,但在实际实现中可能需要根据具体的链表设计调整。
阅读全文
相关推荐
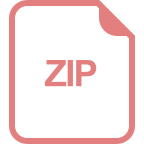
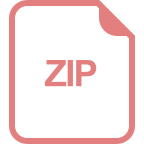
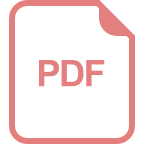















