C语言输入一个四位数,将其加密后输出。方法是将该数每一位上的数字加 9,然后除以 10 取余,做为该位上的新数字,最后将干位和十位上的数字互换,百位和个位上的数字互换,组成加密后的新四位数。例如输入1257,经过加9取余后得到新数字 0146,再经过两次换位后得到 4601
时间: 2024-12-23 22:26:27 浏览: 10
在C语言中,你可以编写如下的程序来实现这个加密过程:
```c
#include <stdio.h>
// 加密函数,接收四位数并返回加密后的四位数
int encrypt(int num) {
int encrypted[4]; // 用于存储加密后的每个数字
int temp = 0;
// 对每位数字加9并取余
for (int i = 0; i < 4; ++i) {
encrypted[i] = (num % 10 + 9) % 10;
num /= 10;
}
// 换位操作
temp = encrypted[0];
encrypted[0] = encrypted[2];
encrypted[2] = temp;
temp = encrypted[1];
encrypted[1] = encrypted[3];
encrypted[3] = temp;
// 将加密后的数字组合成一个新的四位数
int result = 0;
for (int i = 0; i < 4; ++i) {
result = result * 10 + encrypted[i];
}
return result;
}
int main() {
int input;
printf("请输入一个四位数:");
scanf("%d", &input);
int encrypted_num = encrypt(input);
printf("加密后的四位数为:%04d\n", encrypted_num);
return 0;
}
```
在这个程序中,`encrypt` 函数首先对输入的四位数每位加上9并取余,然后交换千位和个位、百位和十位的值。最后将处理过的数字重新组合成一个新的四位数。
阅读全文
相关推荐
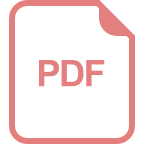
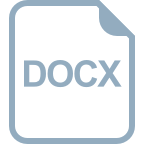
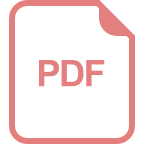








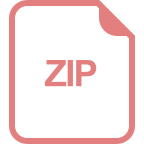
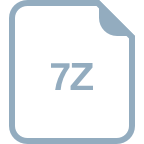