vue3子组件暴露给父元素
时间: 2023-09-28 14:12:38 浏览: 168
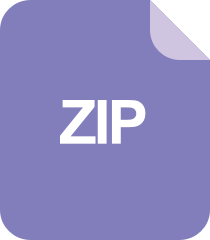
前端VUE3视频教程03
Vue3的子组件可以通过使用`emit`方法将数据暴露给父元素。在子组件的`setup`函数中,可以定义一个方法,例如`update`,用于向父组件发送数据。使用`emit`方法,将指定的事件名和数据作为参数传递,以便父组件能够接收到子组件传递的数据。通过这种方式,子组件可以将数据传递给父元素。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Vue3 - 组件通信(子传父)](https://blog.csdn.net/weixin_44198965/article/details/127875451)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
阅读全文
相关推荐
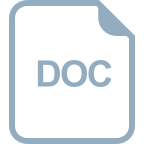
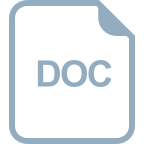
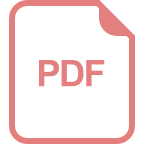



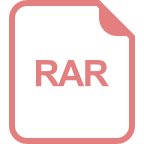
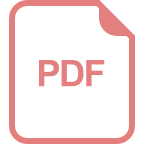
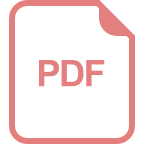



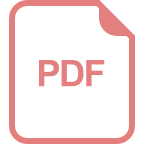
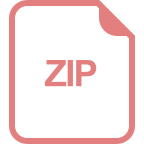
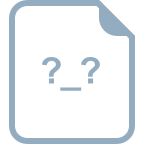
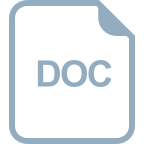
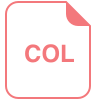
