c语言程序利用队列打印杨辉三角
时间: 2024-11-05 16:09:51 浏览: 35
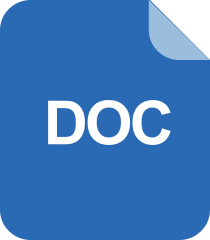
C语言利用队列打印杨辉三角
C语言程序可以使用队列数据结构来打印杨辉三角,这是因为杨辉三角的每一行都是前一行元素的加法组合,这非常适合通过迭代的方式生成。下面是一个简单的步骤说明:
1. 定义一个队列来存储当前行的数字,初始化为第一行的两个1。
2. 创建一个for循环,遍历从0到n-1(n是要打印的层数),对每层i:
- 对于每一层,先将上一行的最后一个元素入队(因为杨辉三角左上角是1,然后每次增加一个1)。
- 然后,从队列头部开始,取出元素并打印,同时依次计算下一个小数(等于当前队列头的值加上下一个位置的值,如果不在队列中,则添加为1),加入队列。
3. 打印完成后,队列为空,循环结束。
这里是一个简单的伪代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 队列结构体和相关的操作函数(假设已经实现)
typedef struct Node {
int value;
struct Node* next;
} QueueNode;
QueueNode* create_queue(int n);
void print_triangle(QueueNode* head, int n);
int main() {
int n = 5; // 想要打印的层数
QueueNode* queue = create_queue(n);
print_triangle(queue, n);
return 0;
}
// 具体实现
QueueNode* create_queue(int n) {
// 初始化队列为[1, 0]
}
void print_triangle(QueueNode* head, int n) {
for (int i = 0; i < n; ++i) {
while (!queue_empty(head)) {
printf("%d ", queue_front_value(head));
enqueue(queue, queue_pop(head)); // 取出并入队下一个数字
}
printf("\n");
}
}
```
阅读全文
相关推荐
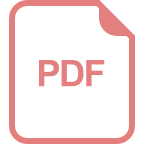
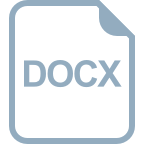





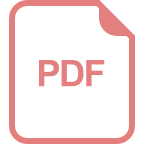
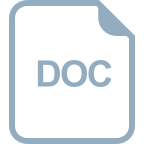








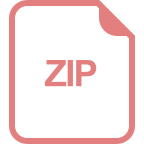