能否提供一个Python实现粒子系统生成动态爱心形状的代码示例?
时间: 2024-12-24 12:35:42 浏览: 7
在Python中,我们可以使用Pygame库创建一个简单的粒子系统,并通过修改粒子的位置、大小和颜色等属性来模拟生成动态爱心形状。这里是一个基础的示例代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口尺寸
win_size = (640, 480)
screen = pygame.display.set_mode(win_size)
pygame.display.set_caption("Particle Heart")
# 粒子类
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.speed = [random.uniform(-5, 5), random.uniform(-5, 5)]
self.size = random.randint(5, 15)
self.color = (255, 0, 0) # 红色
def update(self):
self.x += self.speed[0]
self.y += self.speed[1]
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.size)
# 创建粒子列表
particles = []
for _ in range(50): # 创建50个粒子
particles.append(Particle(random.randint(0, win_size[0]), random.randint(0, win_size[1])))
# 游戏主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255)) # 清除屏幕背景
# 更新和绘制粒子
for particle in particles:
particle.update()
particle.draw()
# 检查粒子是否超出边界,若超出则删除并创建新粒子
particles = [p for p in particles if p.x > 0 and p.x < win_size[0] and p.y > 0 and p.y < win_size[1]]
pygame.display.flip() # 刷新显示
# 结束程序
pygame.quit()
```
这个示例会创建一个红色爱心形状的粒子效果,粒子随机地移动并在屏幕上消失。你可以根据需要调整粒子的颜色、速度和数量。
阅读全文
相关推荐
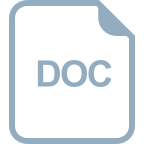
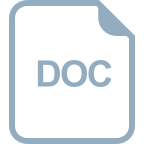
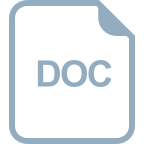







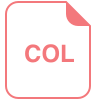
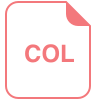
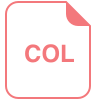
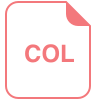
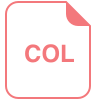
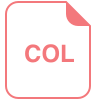
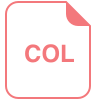
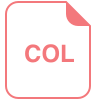
