学生成绩表包括学号,姓名,数学成绩,英语成绩,平均成绩等字段。建立一个顺序表表示学生成绩表,请编写程序,实现以下任务,输入学号,姓名,数学和英语成绩,直到输入学号为0结束
时间: 2024-09-18 11:01:49 浏览: 37
为了实现这个需求,我们可以使用结构体定义学生成绩,并创建一个动态数组(顺序表)来存储这些记录。首先,我们需要定义一个`Student`结构体,然后编写一个主函数来读取学生的数据并插入到数组中。当输入学号为0时,我们停止读取。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义学生成绩结构体
typedef struct {
int id;
char name[50];
float math_score;
float english_score;
float average_score; // 平均成绩默认初始化为0
} Student;
// 动态分配内存并添加新学生到数组
void add_student(Student** students, size_t* size, int id, const char* name, float math, float english) {
if (*size >= 100) { // 如果数组已满,扩展数组容量
*size *= 2;
Student* new_students = realloc(*students, sizeof(Student)*(*size));
if (new_students == NULL) {
printf("Memory allocation failed.\n");
exit(1);
}
*students = new_students;
}
Student* current = &(*students)[*size - 1]; // 获取最后一个元素的地址
current->id = id;
strncpy(current->name, name, sizeof(current->name) - 1); // 注意字符串复制要避免缓冲区溢出
current->math_score = math;
current->english_score = english;
current->average_score = (current->math_score + current->english_score) / 2; // 初次计算平均分
(*size)++;
}
int main() {
Student* students = malloc(sizeof(Student)); // 初始化大小为1
size_t size = 1;
while (true) {
int id;
char name[50];
float math, english;
printf("Enter student information (id 0 to stop): \n");
scanf("%d", &id);
getchar(); // 清除回车符,防止后续输入干扰
if (id == 0)
break;
scanf("%s %f %f", name, &math, &english);
add_student(&students, &size, id, name, math, english);
}
printf("Student records added:\n");
for (int i = 0; i < size; i++) {
printf("ID: %d, Name: %s, Math Score: %.2f, English Score: %.2f, Average Score: %.2f\n",
students[i].id, students[i].name, students[i].math_score, students[i].english_score,
students[i].average_score);
}
free(students); // 释放内存
return 0;
}
```
在这个程序中,我们使用了`malloc`和`realloc`进行动态内存管理。如果需要删除或修改数组中的某个元素,通常还会需要关联一个额外的数据结构来跟踪元素的位置,这里简化了演示,假设数组元素按顺序排列。
阅读全文
相关推荐
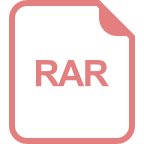
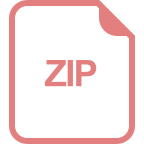
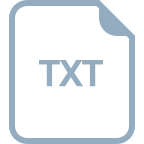













