创建Student类、t_student数据表。 2.创建mybatis框架连接数据库的工具类。 3.创建StudentMapper.xml映射文件。
时间: 2024-10-13 20:07:11 浏览: 30
1. 创建`Student`类通常是一个简单的Java类,它代表数据库中的`t_student`数据表的一个实体。例如,如果`t_student`表包含字段如id、name和age,`Student`类可能会这样定义:
```java
public class Student {
private Integer id;
private String name;
private Integer age;
// 构造函数,getters和setters
public Student(Integer id, String name, Integer age) {
this.id = id;
this.name = name;
this.age = age;
}
// getter和setter方法
public Integer getId() { return id; }
public void setId(Integer id) { this.id = id; }
// 类似的getter和setter方法...
}
```
2. 创建MyBatis框架连接数据库的工具类,可以包括DataSource的配置,以及基本的SqlSessionFactory的初始化方法:
```java
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
public class MyBatisUtil {
private static SqlSessionFactory sqlSessionFactory;
static {
SqlSessionFactoryBean factoryBean = new SqlSessionFactoryBean();
factoryBean.setDataSource(yourDataSource); // 你需要替换为实际的数据源
try {
sqlSessionFactory = factoryBean.getObject();
} catch (Exception e) {
e.printStackTrace();
}
}
public static SqlSessionFactory getSqlSessionFactory() {
return sqlSessionFactory;
}
}
```
注意:这里的`yourDataSource`需要你自己提供,通常是通过Spring IoC容器管理的。
3. `StudentMapper.xml`映射文件用于描述SQL查询如何与Java对象对应。比如一个添加学生的基本映射可能像这样:
```xml
<mapper namespace="com.example.StudentMapper">
<insert id="addStudent" parameterType="com.example.Student">
INSERT INTO t_student (name, age)
VALUES (#{name}, #{age})
</insert>
</mapper>
```
在这个例子中,`namespace`指定了这个映射文件的根包名,`insert`标签定义了一个插入操作,`parameterType`指定传入的参数类型为`Student`对象。
阅读全文
相关推荐
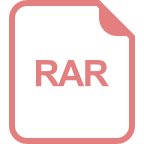
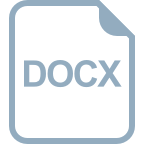
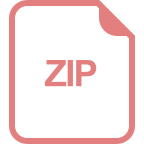















