C++(1)设计一个顺序栈的基本操作的演示程序 (2)设计一个链栈的基本操作的演示程序 (3)设计一个循环队列的基本操作的演示程序 (4)设计一个链队列的基本操作的演示程序
时间: 2024-12-02 22:16:11 浏览: 16
(1) 设计一个顺序栈(数组实现)的基本操作演示程序:
```cpp
#include <iostream>
// 定义栈结构体,包含数据元素和大小,以及栈顶指针
struct Stack {
int capacity;
int* elements;
int top;
};
// 初始化栈
void init(Stack& s, int size) {
s.capacity = size;
s.elements = new int[size];
s.top = -1;
}
// 入栈操作
void push(Stack& s, int value) {
if (s.top == s.capacity - 1) {
std::cout << "Stack overflow!" << std::endl;
return;
}
s.elements[++s.top] = value;
}
// 出栈操作
int pop(Stack& s) {
if (s.top == -1) {
std::cout << "Stack underflow!" << std::endl;
return -1; // 返回一个特殊值表示栈空
}
return s.elements[s.top--];
}
// 检查栈是否为空
bool isEmpty(Stack& s) {
return s.top == -1;
}
int main() {
Stack stack;
init(stack, 5);
push(stack, 10);
push(stack, 20);
push(stack, 30);
while (!isEmpty(stack)) {
std::cout << "Popped element: " << pop(stack) << std::endl;
}
delete[] stack.elements;
return 0;
}
```
(2) 设计一个链栈(链表实现)的基本操作演示程序:
```cpp
#include <iostream>
using namespace std;
// 链栈节点
class Node {
public:
int data;
Node* next;
Node(int d) : data(d), next(nullptr) {}
};
// 链栈类
class LinkedListStack {
private:
Node* top;
public:
void push(int value) {
Node* newNode = new Node(value);
newNode->next = top;
top = newNode;
}
int pop() {
if (top == nullptr) {
cout << "Stack is empty!" << endl;
return -1;
}
int poppedValue = top->data;
Node* temp = top;
top = top->next;
delete temp;
return poppedValue;
}
bool isEmpty() {
return top == nullptr;
}
};
int main() {
LinkedListStack stack;
stack.push(10);
stack.push(20);
stack.push(30);
while (!stack.isEmpty()) {
cout << "Popped element: " << stack.pop() << endl;
}
return 0;
}
```
(3) 设计一个循环队列的基本操作演示程序:
```cpp
#include <iostream>
template<int Size>
class CircularQueue {
private:
int front, rear;
int count;
int buffer[Size];
public:
CircularQueue() : front(-1), rear(-1), count(0) {}
bool enqueue(int value) {
if ((rear + 1) % Size == front) { // 队满
cout << "Queue is full!" << endl;
return false;
}
rear = (rear + 1) % Size;
buffer[rear] = value;
++count;
return true;
}
bool dequeue() {
if (front == rear) { // 队空
cout << "Queue is empty!" << endl;
return false;
}
int dequeuedValue = buffer[front];
front = (front + 1) % Size;
--count;
return true;
}
bool isEmpty() {
return count == 0;
}
};
int main() {
CircularQueue<5> queue;
queue.enqueue(10);
queue.enqueue(20);
queue.enqueue(30);
while (!queue.isEmpty()) {
cout << "Dequeued element: " << queue.dequeue() << endl;
}
return 0;
}
```
(4) 设计一个链队列(双向链表实现)的基本操作演示程序:
```cpp
#include <iostream>
class Node {
public:
int data;
Node* prev;
Node* next;
Node(int d) : data(d), prev(nullptr), next(nullptr) {}
};
class DoublyLinkedListQueue {
private:
Node* front, *rear;
public:
DoublyLinkedListQueue() : front(nullptr), rear(nullptr) {}
void enqueue(int value) {
Node* newNode = new Node(value);
if (rear == nullptr) {
front = rear = newNode;
} else {
newNode->prev = rear;
rear->next = newNode;
rear = newNode;
}
}
void dequeue() {
if (front == nullptr) { // 队空
cout << "Queue is empty!" << endl;
return;
}
Node* removedNode = front;
front = front->next;
if (front != nullptr) front->prev = nullptr;
delete removedNode;
}
bool isEmpty() {
return front == nullptr;
}
};
int main() {
DoublyLinkedListQueue queue;
queue.enqueue(10);
queue.enqueue(20);
queue.enqueue(30);
while (!queue.isEmpty()) {
cout << "Dequeued element: " << queue.dequeue() << endl;
}
return 0;
}
```
阅读全文
相关推荐
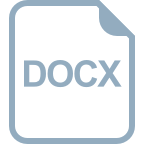
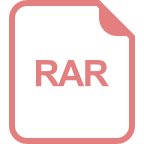
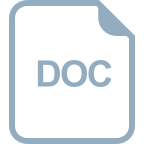
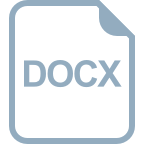
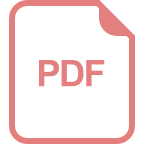
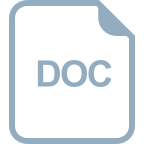
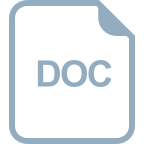
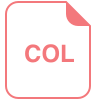
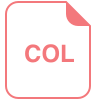


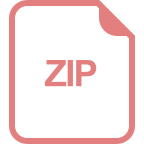
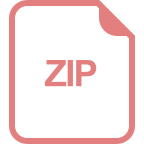
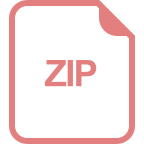
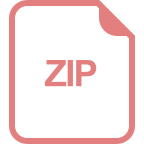
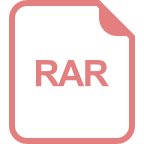
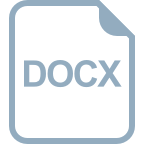
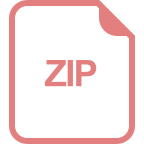