c# 利用freesql如何查询MySQL数据库一年内的所有数据
时间: 2024-04-15 13:29:30 浏览: 16
你可以使用 FreeSql 库来查询 MySQL 数据库的一年内的所有数据。下面是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
using FreeSql;
using FreeSql.DataAnnotations;
public class Program
{
static void Main()
{
var connectionString = "your_connection_string"; // 替换为你的数据库连接字符串
var fsql = new FreeSql.FreeSqlBuilder()
.UseConnectionString(FreeSql.DataType.MySql, connectionString)
.UseAutoSyncStructure(false)
.Build();
// 定义实体类
[Table(Name = "your_table_name")] // 替换为你的表名
class YourEntity
{
[Column(IsPrimary = true)]
public int Id { get; set; }
public DateTime Date { get; set; }
// 其他字段...
}
// 查询一年内的数据
var oneYearAgo = DateTime.Now.AddYears(-1);
var list = fsql.Select<YourEntity>().Where(e => e.Date >= oneYearAgo).ToList();
foreach (var item in list)
{
Console.WriteLine($"Id: {item.Id}, Date: {item.Date}");
// 处理其他字段...
}
}
}
```
在代码中,你需要将 `your_connection_string` 替换为自己的数据库连接字符串,将 `your_table_name` 替换为你要查询的表名,以及定义对应的实体类。
这样,你就可以使用 FreeSql 进行 MySQL 数据库的查询了。注意,这里的示例代码是基于 FreeSql v3.2.2 版本编写的,如果你使用了其他版本,可能会有一些差异。你可以根据自己的实际情况进行调整。
相关推荐
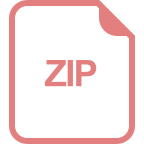
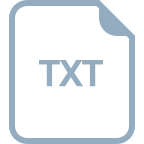
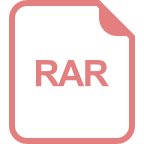














