STM32 printf
时间: 2024-12-25 20:18:17 浏览: 2
STM32微控制器通常使用标准的ANSI C库函数printf来进行输出,但这并不是直接内置的功能,而是需要通过配置硬件串口、USB虚拟COM端口或者其他通信接口,并配合相应的驱动和中间件来实现。在STM32上,最常用的是使用HAL(Hardware Abstraction Layer,硬件抽象层)提供的功能,它封装了底层的硬件操作,允许用户像在PC上那样使用printf。
首先,你需要初始化相关的串口或者USART(通用异步收发器),然后设置好printf格式化字符串的输出目标。例如,你可以这样使用:
```c
#include "stm32f1xx_hal.h"
#include <stdio.h>
void USART_Printf(USART_HandleTypeDef *husart, const char* format, ...) {
va_list args;
va_start(args, format);
HAL_UART_Transmit(&husart->Instance, (uint8_t*)format, strlen(format), HAL_MAX_DELAY);
vprintf((char*)format, args);
va_end(args);
}
```
在这个例子中,`USART_Printf`函数接收一个USART句柄和一个printf格式化的字符串,它会先发送字符串,然后再传递给vprintf处理变量参数。
相关问题
stm32 printf
The STM32 microcontroller supports the printf() function from the C standard library. This function is used to output formatted text to a console or other output device.
To use printf() on an STM32 microcontroller, you first need to initialize the USART peripheral and configure it for the desired baud rate and other settings. Then, you can use the printf() function in your code to output text to the USART.
Here is an example code snippet that demonstrates how to use printf() on an STM32 microcontroller:
```
#include <stdio.h>
#include "stm32f4xx.h"
int main(void)
{
// Initialize USART2 peripheral
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART2, &USART_InitStructure);
USART_Cmd(USART2, ENABLE);
// Use printf to output text to USART2
printf("Hello, world!\r\n");
while (1)
{
// do other stuff here
}
}
```
In this example, we first initialize the USART2 peripheral with the desired settings. Then, we use printf() to output the text "Hello, world!" to the USART. Finally, we enter an infinite loop to keep the microcontroller running.
Note that in order to use printf() on an STM32 microcontroller, you need to have a working implementation of the stdio library. This can be included as part of your project or provided by the vendor of your development board or toolchain.
stm32printf
在STM32上使用printf函数输出信息需要进行重定向。通过重定向,可以将printf函数的输出发送到仿真器连接的PC机上的终端窗口。具体实现方法如下:
1. 首先,需要在工程中添加一个文件,命名为retarget.c,并将其加入到工程中。
2. 在retarget.c文件中,需要实现fputc函数,该函数将会被printf函数调用。在fputc函数中,可以利用ITM(Instrumentation Trace Macrocell)机制提供的寄存器,将数据发送给仿真器,然后仿真器将数据发送到PC机上的终端窗口。以下是一个示例的fputc函数的实现:
```c
#include <stdio.h>
#define ITM_Port8(n) (*((volatile unsigned char *)(0xE0000000 + 4*n)))
#define ITM_Port16(n) (*((volatile unsigned short *)(0xE0000000 + 4*n)))
#define ITM_Port32(n) (*((volatile unsigned long *)(0xE0000000 + 4*n)))
#define DEMCR (*((volatile unsigned long *)(0xE000EDFC)))
#define TRCENA 0x01000000
struct __FILE { int handle; /* Add whatever you need here */ };
FILE __stdout;
FILE __stdin;
int fputc(int ch, FILE *f) {
if (DEMCR & TRCENA) {
while (ITM_Port32(0) == 0);
ITM_Port8(0) = ch;
}
return ch;
}
```
3. 在工程中的main函数中,需要添加以下代码来重定向printf函数的输出:
```c
#include <stdio.h>
extern void initialise_monitor_handles(void);
int main() {
initialise_monitor_handles();
// 其他代码
printf("Hello, world!");
// 其他代码
return 0;
}
```
通过以上步骤,就可以在STM32上使用printf函数并将输出重定向到仿真器连接的PC机上的终端窗口了。
阅读全文
相关推荐
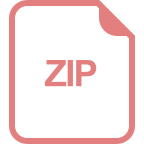
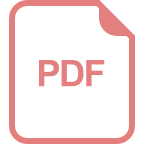
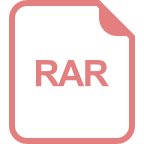













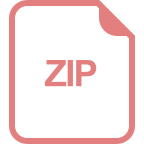