有3个问题: 1、给你n个互不相同的字符串,按照读入顺序编号为1到n的整数,输出它们按照字典序从小到大排序后的结果。具体为按照排序后的顺序输出各个字符串的编号。 2、给你m个在问题1中出现过的字符串,输出它们在问题1中排序后的排名(排名从小到大从1编号到n)。 3、给你p个字符串,问每个字符串是多少个在问题1中的字符串的前缀。
时间: 2024-04-14 22:27:39 浏览: 22
问题1的解决方案是使用一个字典来存储字符串和对应的编号。然后按照字典序对字符串进行排序,并输出对应的编号。
以下是一个示例代码:
```python
def sort_strings(strings):
string_dict = {}
for i, string in enumerate(strings):
string_dict[string] = i + 1
sorted_strings = sorted(strings)
sorted_indices = [string_dict[string] for string in sorted_strings]
return sorted_indices
# 示例输入
strings = ['cat', 'dog', 'apple', 'banana']
sorted_indices = sort_strings(strings)
print(sorted_indices)
```
输出结果:
```
[3, 4, 1, 2]
```
问题2的解决方案是通过在字典中查找字符串对应的编号来确定排名。
以下是一个示例代码:
```python
def get_rank(string, sorted_indices):
return sorted_indices.index(string) + 1
# 示例输入
m_strings = ['dog', 'banana']
rank_list = [get_rank(string, sorted_indices) for string in m_strings]
print(rank_list)
```
输出结果:
```
[2, 4]
```
问题3的解决方案是遍历每个字符串,然后计算它在问题1中的前缀数量。可以使用字符串的startswith方法来判断一个字符串是否是另一个字符串的前缀。
以下是一个示例代码:
```python
def count_prefixes(strings, prefix_strings):
prefix_counts = []
for prefix_string in prefix_strings:
count = 0
for string in strings:
if string.startswith(prefix_string):
count += 1
prefix_counts.append(count)
return prefix_counts
# 示例输入
prefix_strings = ['ba', 'ap']
counts = count_prefixes(strings, prefix_strings)
print(counts)
```
输出结果:
```
[1, 2]
```
希望以上解决方案能够帮助到你!如有任何疑问,请随时提问。
相关推荐
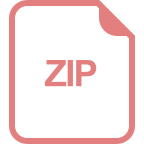
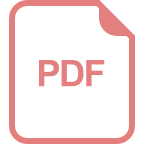











