编写程序实现计算长方体表面积和体积:从键盘输入长=12.0,宽=4.0,高=6.0,输出其表面积和体积
时间: 2024-06-12 16:09:11 浏览: 21
您可以通过编写一个程序来计算长方体的表面积和体积。首先,您需要定义一个抽象类Shape,并从该类派生出长方体类。在长方体类中,您可以实现继承的纯虚函数以计算表面积和体积。
以下是一个示例程序的框架:
```
#include<iostream>
using namespace std;
// 抽象类Shape
class Shape {
public:
// 纯虚函数,用于计算表面积
virtual double calculateArea() = 0;
// 纯虚函数,用于计算体积
virtual double calculateVolume() = 0;
};
// 长方体类,继承自Shape类
class Cuboid : public Shape {
private:
double length;
double width;
double height;
public:
// 构造函数
Cuboid(double l, double w, double h) : length(l), width(w), height(h) {}
// 实现继承的纯虚函数,计算表面积
double calculateArea() {
return 2 * (length * width + length * height + width * height);
}
// 实现继承的纯虚函数,计算体积
double calculateVolume() {
return length * width * height; }
};
int main() {
// 从键盘输入长、宽、高
double length, width, height;
cout << "请输入长方体的长、宽和高:";
cin >> length >> width >> height;
// 创建长方体对象
Cuboid cuboid(length, width, height);
// 计算表面积和体积
double area = cuboid.calculateArea();
double volume = cuboid.calculateVolume();
// 输出结果
cout << "长方体的表面积为:" << area << endl;
cout << "长方体的体积为:" << volume << endl;
return 0;
}
```
以上程序可以实现从键盘输入长方体的长、宽和高,然后计算并输出其表面积和体积。
相关推荐
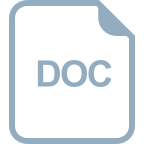
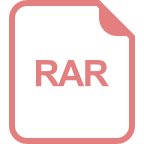
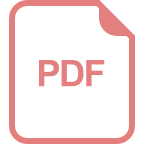














