使用c语言程序实现数据结构删除单链表中所有节点
时间: 2024-10-24 09:07:22 浏览: 43
在C语言中,删除单链表中所有节点通常涉及以下几个步骤:
1. 定义链表结构:首先,我们需要定义一个`struct Node`表示链表中的一个节点,包含一个`data`元素和一个指向下一个节点的指针`next`。
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 初始化链表:创建链表时,我们通常会有一个头结点`head`,初始化时设置为NULL。
```c
Node* head = NULL;
```
3. 删除节点:遍历链表,对于每个节点,将其值赋给`current`,然后将`current->next`设为`current->next->next`,即跳过当前节点。最后更新`head`为新的头结点,直到遍历完整个链表。
```c
void deleteList(Node** head) {
while (*head != NULL) {
Node* temp = *head;
*head = (*head)->next; // 将头结点移向下一个
free(temp); // 释放当前节点内存
}
}
```
4. 示例完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 删除链表函数
void deleteList(Node** head);
int main() {
// 创建并初始化链表示例
Node* list = (Node*)malloc(sizeof(Node));
list->data = 1;
list->next = (Node*)malloc(sizeof(Node));
list->next->data = 2;
list->next->next = NULL;
printf("Original List: ");
printList(list);
deleteList(&list);
printf("\nList after deletion: ");
if (list == NULL) {
printf("Empty\n");
} else {
printf("Not deleted properly! [This should not happen]\n");
}
return 0;
}
// 打印链表函数
void printList(Node* node) {
while (node != NULL) {
printf("%d -> ", node->data);
node = node->next;
}
printf("NULL\n");
}
// 删除链表函数
void deleteList(Node** head) {
while (*head != NULL) {
Node* temp = *head;
*head = (*head)->next; // 将头结点移向下一个
free(temp); // 释放当前节点
}
}
```
阅读全文
相关推荐
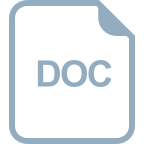
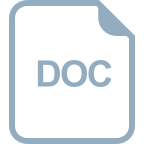
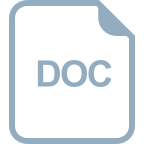

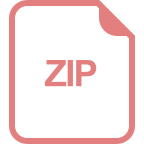
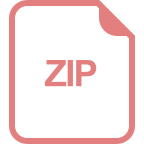
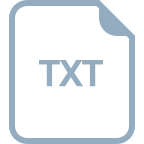
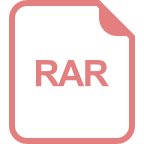
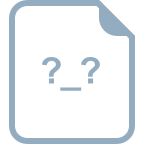
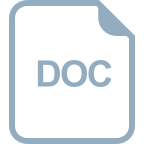
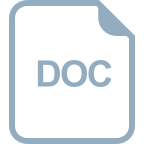






