用C++的引用,输入若干学生信息,分别按照姓名顺序,总成绩顺序,从小到大输出包括学号,姓名,科目(语文,数学,英语)
时间: 2024-09-19 16:03:44 浏览: 35
在C++中,我们可以创建一个结构体(struct)来存储学生的相关信息,然后使用引用和容器(如vector或list)来管理学生数据。首先,我们需要定义一个名为`Student`的结构体,包含学号、姓名和各科成绩。这里是一个简单的例子:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm> // 使用sort函数
// 定义学生结构体
struct Student {
int id;
std::string name;
int chinese, math, english;
};
// 比较函数,用于排序
bool compareByName(const Student& a, const Student& b) {
return a.name < b.name;
}
bool compareByTotalScore(const Student& a, const Student& b) {
return (a.chinese + a.math + a.english) < (b.chinese + b.math + b.english);
}
int main() {
std::vector<Student> students; // 存储学生信息
std::string name, input;
// 输入学生信息
while (true) {
std::cout << "请输入学生信息(格式:学号 姓名 数学成绩 语文成绩 英语成绩,输入'q'退出):" << std::endl;
std::cin >> name >> input;
if (input == "q") break;
// 将输入分割并转换为整数
int values[] = {0, 0, 0};
for (size_t i = 0; i < 3; ++i) {
std::istringstream iss(input);
iss >> values[i];
}
students.push_back({values[0], name, values[1], values[2]});
}
// 按照姓名排序
std::sort(students.begin(), students.end(), compareByName);
// 输出按姓名排序的学生信息
std::cout << "按姓名排序:" << std::endl;
for (const auto& student : students) {
std::cout << "学号:" << student.id << ", 姓名:" << student.name << ", 成绩:语文(" << student.chinese << ") 数学(" << student.math << ") 英语(" << student.english << ")" << std::endl;
}
// 按总分排序
std::sort(students.begin(), students.end(), compareByTotalScore);
// 输出按总分排序的学生信息
std::cout << "按总分排序:" << std::endl;
for (const auto& student : students) {
std::cout << "学号:" << student.id << ", 姓名:" << student.name << ", 成绩:语文(" << student.chinese << ") 数学(" << student.math << ") 英语(" << student.english << ")" << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
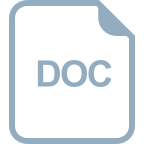
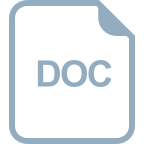
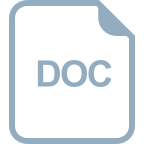




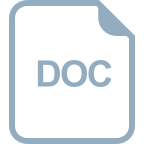
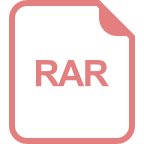
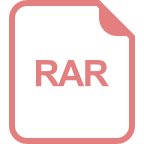
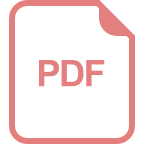
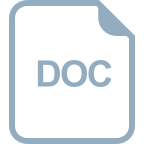
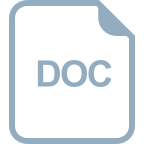
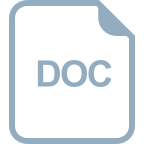
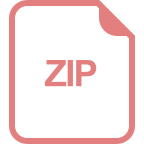
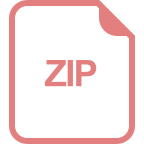
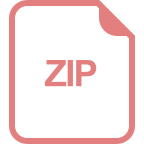
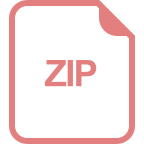