pta题库答案python 求π的近似值
时间: 2024-10-10 20:03:53 浏览: 60
在Python中,计算圆周率π的近似值通常会用到数学公式或者一些常见的算法,比如Leibniz公式、Chudnovsky算法等。其中最简单的一个方法就是使用马青公式(Monte Carlo方法)通过模拟随机点落在单位圆内的概率来估算π。
这是一个基于随机抽样的例子:
```python
import random
def estimate_pi(n):
num_points_circle = 0
total_points = n * n
for _ in range(total_points):
x = random.uniform(0, 1)
y = random.uniform(0, 1)
if x**2 + y**2 <= 1:
num_points_circle += 1
pi_approximation = 4 * (num_points_circle / total_points)
return pi_approximation
# 示例,计算1000000个点下的π估计值
estimate = estimate_pi(1000000)
print(f"使用1000000个点的随机采样,π的估计值大约是 {estimate}")
相关问题
pta题库答案python成绩统计
Python成绩统计通常指的是通过编程实现对一组Python课程成绩数据的收集、处理和分析的过程。这可能包括输入成绩、计算平均分、统计最高分和最低分、计算不同分数段的人数等。对于pta(Programming Teaching Assistant,编程教学辅助系统)题库中的答案和成绩统计,一般会通过编写Python脚本来完成。以下是一个简单的例子来说明如何进行成绩统计:
```python
# 假设这是从pta题库获取的成绩列表
scores = [92, 85, 78, 67, 90, 88, 76, 84, 65, 58]
# 计算平均分
average_score = sum(scores) / len(scores)
# 找出最高分和最低分
highest_score = max(scores)
lowest_score = min(scores)
# 统计不同分数段的人数
score_distribution = {100: 0, 90: 0, 80: 0, 70: 0, 60: 0, 50: 0, 0: 0} # 初始化分数段字典
for score in scores:
if score == 100:
score_distribution[100] += 1
elif score >= 90:
score_distribution[90] += 1
elif score >= 80:
score_distribution[80] += 1
elif score >= 70:
score_distribution[70] += 1
elif score >= 60:
score_distribution[60] += 1
elif score >= 50:
score_distribution[50] += 1
else:
score_distribution[0] += 1
# 输出统计结果
print(f"平均分:{average_score}")
print(f"最高分:{highest_score}")
print(f"最低分:{lowest_score}")
print("分数段统计:")
for score, count in score_distribution.items():
if score != 0:
print(f" {score}分段的人数:{count}")
```
这个脚本首先计算了平均分,然后找出了最高分和最低分,并且统计了每个分数段的人数。这只是一个非常基础的例子,实际的成绩统计可能会更加复杂,包括成绩的输入验证、异常处理、格式化输出、数据持久化等功能。
pta题库答案python 素数排位
pta题库答案Python素数排位是指解决某些在线编程挑战(如Pascal's Triangle of Primes)中关于素数排列的问题。在这个问题中,你需要生成帕斯卡三角形(一种数学图形),其中每个数字要么是一个素数,要么是它上方两个素数的乘积。例如,对于给定的高度,你需要计算并显示包含适当素数的帕斯卡三角形。
Python中实现这个功能的一个常见做法是使用`itertools.combinations()`函数生成可能的组合,并通过检查每个数是否为素数来填充三角形。你可以利用Python的标准库函数如`isprime()`来自`math`模块来判断一个数是否为素数。
下面是一个简化的示例代码片段:
```python
import itertools
from math import isqrt
def is_prime(n):
if n <= 1:
return False
for i in range(2, isqrt(n) + 1):
if n % i == 0:
return False
return True
def prime_pascals_triangle(height):
triangle = [[1]]
primes = [2] # 初始化第一个素数位置
for _ in range(1, height):
new_row = []
for combination in itertools.permutations(primes, r=2): # 取前两个素数组合
product = combination[0] * combination[1]
if is_prime(product):
new_row.append(product)
primes.extend(new_row) # 更新当前行后的素数列表
triangle.append(new_row)
return triangle
height = int(input("请输入帕斯卡三角形的高度:"))
result = prime_pascals_triangle(height)
for row in result:
print(row)
阅读全文
相关推荐
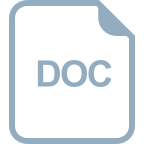
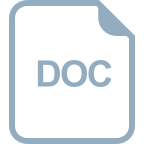
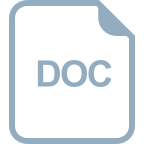
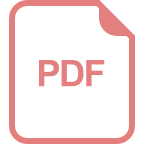






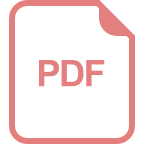


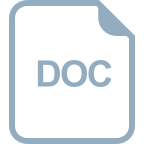