请编写一段C++程序,利用opencv分别在两个线程中完成图像的灰度化和旋转
时间: 2024-04-12 08:29:20 浏览: 114
下面是一段使用 OpenCV 在两个线程中进行图像灰度化和旋转的 C++ 代码示例:
```cpp
#include <iostream>
#include <thread>
#include <opencv2/opencv.hpp>
void grayscale(cv::Mat& image) {
cv::cvtColor(image, image, cv::COLOR_BGR2GRAY);
}
void rotate(cv::Mat& image, double angle) {
cv::Point2f center(image.cols / 2.0, image.rows / 2.0);
cv::Mat rotationMatrix = cv::getRotationMatrix2D(center, angle, 1.0);
cv::warpAffine(image, image, rotationMatrix, image.size());
}
int main() {
cv::Mat image = cv::imread("image.jpg");
if (image.empty()) {
std::cout << "Failed to read image" << std::endl;
return -1;
}
// 创建两个线程分别进行灰度化和旋转
std::thread thread1(grayscale, std::ref(image));
std::thread thread2(rotate, std::ref(image), 45.0);
// 等待两个线程执行结束
thread1.join();
thread2.join();
// 显示结果
cv::imshow("Result", image);
cv::waitKey(0);
return 0;
}
```
在上述代码中,`grayscale` 函数将图像转换为灰度图像,`rotate` 函数将图像按照指定角度进行旋转。在 `main` 函数中,我们首先读取一张图像,并创建两个线程分别调用 `grayscale` 和 `rotate` 函数对图像进行处理。然后,使用 `thread1.join()` 和 `thread2.join()` 等待两个线程执行结束。最后,显示处理后的图像。
请确保已经安装了 OpenCV 库,并将图像文件命名为 "image.jpg",与代码放在同一目录下。你也可以根据自己的需要修改代码,例如调整图像处理函数的参数或添加更多的线程来执行其他任务。
阅读全文
相关推荐
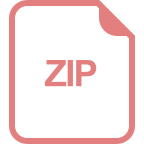
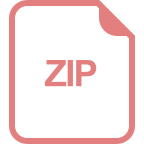
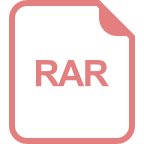
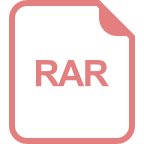
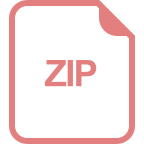
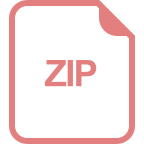
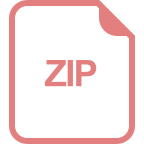
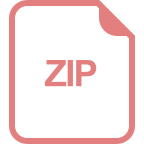
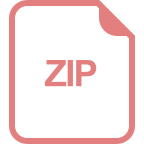
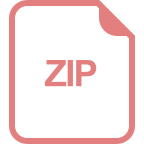
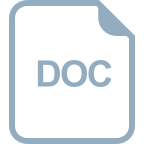
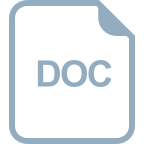





