python求二维数组的长度
时间: 2023-08-16 22:13:38 浏览: 253
你可以使用 Python 的内置函数 `len()` 来求二维数组的长度。二维数组实际上是一个包含多个子数组的列表,所以你可以先通过索引获取其中一个子数组,然后再使用 `len()` 函数求其长度。下面是一个示例:
```python
array_2d = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
length = len(array_2d[0]) # 获取第一个子数组的长度
print(length) # 输出:3
```
在上面的示例中,`array_2d` 是一个包含三个子数组的二维数组。我们通过索引 `array_2d[0]` 获取第一个子数组,然后使用 `len()` 函数求其长度。最终结果为 3,表示第一个子数组的长度。
相关问题
python txt 二维数组
### 回答1:
Python中可以使用文本文件(txt)来表示和处理二维数组。下面是一个使用txt文件来表示二维数组的示例:
首先,我们可以创建一个txt文件,例如"array.txt",用来存储二维数组的数据。在该文件中,可以使用逗号作为分隔符来分割不同的元素,并用换行符来分割不同的行:
```plaintext
1,2,3
4,5,6
7,8,9
```
然后,可以使用Python的文件读取功能来读取该txt文件,并将其转换为二维数组。例如,可以使用以下代码将"array.txt"中的数据读取并存储到一个二维数组中:
```python
array = []
with open("array.txt", "r") as file:
for line in file:
row = [int(value) for value in line.strip().split(",")]
array.append(row)
```
上述代码将逐行读取文件内容,然后使用split函数将每行的数据按逗号分割为不同的元素,并使用int函数将其转换为整数。然后,将每一行转换后的列表作为一个行添加到二维数组中。
现在,我们可以使用array变量来访问和操作该二维数组的元素。例如,可以使用下标来访问特定的行和列:
```python
print(array[0][0]) # 输出第一行第一列的值
print(array[1][2]) # 输出第二行第三列的值
```
除了这些基本的操作,Python还提供了许多用于处理二维数组的函数和方法,例如计算数组的长度、遍历数组的所有元素、对数组进行排序等。
总结起来,使用文本文件和Python的文件读取功能,可以很方便地将二维数组表示为txt文件,并通过读取文件并转换为二维数组来使用和操作它们。
### 回答2:
Python中可以使用txt文件来表示二维数组。具体实现的步骤如下:
1. 创建一个txt文件,可以使用open函数来创建文件对象,设置文件名和操作模式为写入模式。
2. 使用循环遍历二维数组,将每个元素写入txt文件中。可以使用文件对象的write方法来写入数据,每个元素之间可以使用空格或其他分隔符分隔。
3. 保存并关闭文件对象,使用文件对象的close方法来关闭文件。
以下是一个示例代码:
```python
# 创建一个二维数组
array = [[1, 2, 3],
[4, 5, 6],
[7, 8, 9]]
# 打开文件并写入数据
with open("array.txt", "w") as file:
for row in array:
for element in row:
# 使用空格分隔每个元素
file.write(str(element) + " ")
# 换行
file.write("\n")
# 关闭文件
file.close()
```
运行上述代码后,将会在当前目录下生成一个名为"array.txt"的文本文件,文件内容如下所示:
```
1 2 3
4 5 6
7 8 9
```
这样,我们就成功将二维数组保存为txt文件了。如果想要读取这个txt文件并重新生成二维数组,可以使用类似的步骤,通过打开文件、逐行读取文件内容以及使用分隔符切割每行数据的方法来实现。
python将二维数组变为一维数组
你可以使用numpy库中的flatten方法将二维数组变为一维数组。例如:
```python
import numpy as np
# 创建一个二维数组
arr_2d = np.array([[1, 2], [3, 4]])
# 使用flatten方法将二维数组变为一维数组
arr_1d = arr_2d.flatten()
print(arr_1d) # 输出 [1 2 3 4]
```
你也可以使用reshape方法将二维数组变为一维数组。例如:
```python
import numpy as np
# 创建一个二维数组
arr_2d = np.array([[1, 2], [3, 4]])
# 使用reshape方法将二维数组变为一维数组
arr_1d = arr_2d.reshape(-1)
print(arr_1d) # 输出 [1 2 3 4]
```
这里的`-1`表示自动计算数组的长度,即根据原始数组的维度来自动计算新的数组长度。
相关推荐
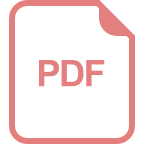
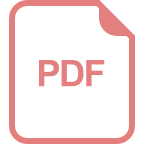
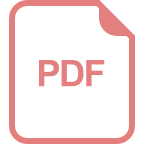












