如何计算两个数A和B的加法,并将其转换为十六进制和十进制在C语言中表示?
时间: 2024-10-19 07:04:47 浏览: 48
在C语言中,你可以使用基本的算术运算符`+`来计算两个整数`A`和`B`的加法。首先,将这两个数相加并存储结果,例如:
```c
int A = 10;
int B = 20;
int sum = A + B; // sum 现在就是 30
```
然后,要将这个结果转换为十六进制,可以使用标准库函数`itoa()`或`sprintf()`配合`%x`格式化字符。注意,`itoa()`在一些较老的系统中可能不可用,这时可以使用其他第三方库或者手动处理。
```c
char hex[10];
itoa(sum, hex, 16); // 使用itoa()将十进制转成十六进制
```
如果使用`sprintf()`,则示例代码如下:
```c
char hex[10];
sprintf(hex, "%x", sum);
```
至于十进制表示,由于`sum`变量本身就是十进制形式,无需额外操作。
如果你需要在程序中显示这些值,可以直接打印`hex`数组和`sum`:
```c
printf("十进制和: %d\n", sum);
printf("十六进制: %s\n", hex);
```
相关问题
C语言中十六进制相加怎么表示
在C语言中,十六进制数的加法通常涉及到字符类型的处理,因为C语言的标准库并没有直接提供对十六进制整数操作的支持。你可以手动将十六进制字符串转换为整数数组,然后逐位相加,最后再转换回十六进制字符串。
以下是一个简单的示例,展示了如何通过ASCII码值实现两个十六进制数字的相加:
```c
#include <stdio.h>
#include <string.h>
char add_hex_chars(char a, char b) {
int sum = a - '0' + b - '0'; // ASCII码减去'0'后的差即为十进制数值
if (sum > 9) { // 十六进制最大只能到F
sum -= 10;
}
return sum + '0';
}
void hex_addition(char* hex1, char* hex2, char* result) {
int len1 = strlen(hex1), len2 = strlen(hex2);
int carry = 0;
for (int i = len1 - 1; i >= 0 || carry; i--) {
if (i < len1) {
carry += hex1[len1 - 1 - i] - '0'; // 右移一位
}
if (i < len2) {
carry += hex2[len2 - 1 - i] - '0';
}
result[i] = add_hex_chars(carry % 16, carry / 16 ? 'A' : '0'); // 对结果取模并转换回十六进制
carry /= 16;
}
result[len1 + len2] = '\0'; // 结果字符串添加终止符
}
int main() {
char hex1[] = "1A";
char hex2[] = "B";
char result[20];
hex_addition(hex1, hex2, result);
printf("Hex Addition: %s\n", result); // 输出结果
return 0;
}
```
这个例子仅支持两位十六进制数的相加,实际应用中可能会需要更复杂的解析和处理机制。记得在实际项目中处理边界情况,并考虑错误检查。
c语言写一个代码,可以实现长整数加减除以及二进制和十六进制转换,除法输出余数和商
以下是一个基本的长整数加减除法的 C 语言代码,同时包括二进制和十六进制转换功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXLEN 1000
// 长整数加法
void add(char* a, char* b, char* result) {
int carry = 0, sum, i = strlen(a) - 1, j = strlen(b) - 1, k = 0;
while (i >= 0 || j >= 0) {
sum = carry + (i >= 0 ? a[i--] - '0' : 0) + (j >= 0 ? b[j--] - '0' : 0);
result[k++] = sum % 10 + '0';
carry = sum / 10;
}
if (carry) result[k++] = carry + '0';
result[k] = '\0';
strrev(result);
}
// 长整数减法
void substract(char* a, char* b, char* result) {
int borrow = 0, diff, i = strlen(a) - 1, j = strlen(b) - 1, k = 0;
while (i >= 0 || j >= 0) {
diff = borrow + (i >= 0 ? a[i--] - '0' : 0) - (j >= 0 ? b[j--] - '0' : 0);
if (diff < 0) {
diff += 10;
borrow = -1;
}
else borrow = 0;
result[k++] = diff + '0';
}
while (k > 1 && result[k - 1] == '0') k--;
result[k] = '\0';
strrev(result);
}
// 长整数除法
void divide(char* a, char* b, char* quotient, char* remainder) {
char temp[MAXLEN];
memset(temp, 0, sizeof(temp));
memset(quotient, 0, sizeof(quotient));
memset(remainder, 0, sizeof(remainder));
int lena = strlen(a), lenb = strlen(b);
if (lena < lenb || (lena == lenb && strcmp(a, b) < 0)) {
strcpy(remainder, a);
return;
}
// 将被除数的前面补0
int k = lena - lenb;
for (int i = 0; i < k; i++) {
temp[i] = '0';
}
for (int i = k; i < lena; i++) {
temp[i] = b[i - k];
}
lena = strlen(a);
lenb = strlen(temp);
temp[lenb] = '\0';
while (k >= 0) {
while (strcmp(a, temp) >= 0) {
substract(a, temp, a);
quotient[k]++;
}
k--;
if (k < 0) break;
memmove(temp + 1, temp, lenb);
lenb++;
temp[0] = '0';
quotient[k] = '0';
}
while (strlen(quotient) > 1 && quotient[0] == '0') {
memmove(quotient, quotient + 1, strlen(quotient));
}
strcpy(remainder, a);
}
// 十进制转二进制
void decimalToBinary(char* decimal, char* binary) {
char temp[MAXLEN];
memset(temp, 0, sizeof(temp));
int n = atoi(decimal), i = 0;
while (n) {
temp[i++] = n % 2 + '0';
n /= 2;
}
if (i == 0) {
strcpy(binary, "0");
}
else {
for (int j = i - 1; j >= 0; j--) {
binary[i - j - 1] = temp[j];
}
binary[i] = '\0';
}
}
// 十进制转十六进制
void decimalToHex(char* decimal, char* hex) {
char temp[MAXLEN];
memset(temp, 0, sizeof(temp));
int n = atoi(decimal), i = 0;
while (n) {
int mod = n % 16;
if (mod < 10) temp[i++] = mod + '0';
else temp[i++] = mod - 10 + 'A';
n /= 16;
}
if (i == 0) {
strcpy(hex, "0");
}
else {
for (int j = i - 1; j >= 0; j--) {
hex[i - j - 1] = temp[j];
}
hex[i] = '\0';
}
}
// 二进制转十进制
void binaryToDecimal(char* binary, char* decimal) {
int n = strlen(binary), sum = 0;
for (int i = 0; i < n; i++) {
sum = sum * 2 + binary[i] - '0';
}
sprintf(decimal, "%d", sum);
}
// 十六进制转十进制
void hexToDecimal(char* hex, char* decimal) {
int n = strlen(hex), sum = 0;
for (int i = 0; i < n; i++) {
if (hex[i] >= '0' && hex[i] <= '9') sum = sum * 16 + hex[i] - '0';
else if (hex[i] >= 'A' && hex[i] <= 'F') sum = sum * 16 + hex[i] - 'A' + 10;
else if (hex[i] >= 'a' && hex[i] <= 'f') sum = sum * 16 + hex[i] - 'a' + 10;
}
sprintf(decimal, "%d", sum);
}
int main() {
char a[MAXLEN], b[MAXLEN], result[MAXLEN], quotient[MAXLEN], remainder[MAXLEN], binary[MAXLEN], hex[MAXLEN];
int choice;
printf("请选择操作:\n1. 长整数加法\n2. 长整数减法\n3. 长整数除法\n4. 十进制转二进制\n5. 十进制转十六进制\n6. 二进制转十进制\n7. 十六进制转十进制\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入两个长整数:\n");
scanf("%s%s", a, b);
add(a, b, result);
printf("结果为:%s\n", result);
break;
case 2:
printf("请输入两个长整数:\n");
scanf("%s%s", a, b);
substract(a, b, result);
printf("结果为:%s\n", result);
break;
case 3:
printf("请输入两个长整数:\n");
scanf("%s%s", a, b);
divide(a, b, quotient, remainder);
printf("商:%s,余数:%s\n", quotient, remainder);
break;
case 4:
printf("请输入一个十进制数:\n");
scanf("%s", a);
decimalToBinary(a, binary);
printf("结果为:%s\n", binary);
break;
case 5:
printf("请输入一个十进制数:\n");
scanf("%s", a);
decimalToHex(a, hex);
printf("结果为:%s\n", hex);
break;
case 6:
printf("请输入一个二进制数:\n");
scanf("%s", binary);
binaryToDecimal(binary, result);
printf("结果为:%s\n", result);
break;
case 7:
printf("请输入一个十六进制数:\n");
scanf("%s", hex);
hexToDecimal(hex, result);
printf("结果为:%s\n", result);
break;
default:
printf("无效的选择!\n");
break;
}
return 0;
}
```
此代码可实现长整数加减除以及二进制和十六进制的转换。
阅读全文
相关推荐
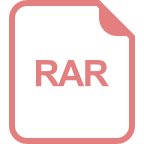















