用C语言实现一个矩阵加法,减法,乘法,数乘,转置的运算的系统
时间: 2024-07-01 17:00:29 浏览: 177
在C语言中,我们可以创建一个简单的矩阵运算系统,使用二维数组来存储矩阵,并定义相应的函数来执行加法、减法、乘法、数乘和转置操作。这里是一个基础的实现示例:
```c
#include <stdio.h>
// 定义矩阵结构体
typedef struct {
int rows;
int cols;
int data[100][100]; // 假设我们处理的矩阵不大于100x100
} Matrix;
// 矩阵加法
Matrix add(Matrix a, Matrix b) {
Matrix result = {a.rows, a.cols};
for (int i = 0; i < result.rows; i++) {
for (int j = 0; j < result.cols; j++) {
result.data[i][j] = a.data[i][j] + b.data[i][j];
}
}
return result;
}
// 矩阵减法
Matrix subtract(Matrix a, Matrix b) {
Matrix result = {a.rows, a.cols};
for (int i = 0; i < result.rows; i++) {
for (int j = 0; j < result.cols; j++) {
result.data[i][j] = a.data[i][j] - b.data[i][j];
}
}
return result;
}
// 矩阵乘法(假设都是方阵)
Matrix multiply(Matrix a, Matrix b) {
if (a.cols != b.rows) {
printf("错误:无法相乘,矩阵列数不匹配\n");
return NULL;
}
Matrix result = {a.rows, b.cols};
for (int i = 0; i < result.rows; i++) {
for (int j = 0; j < result.cols; j++) {
int sum = 0;
for (int k = 0; k < a.cols; k++) {
sum += a.data[i][k] * b.data[k][j];
}
result.data[i][j] = sum;
}
}
return result;
}
// 数字乘矩阵
Matrix numMultiply(int num, Matrix a) {
Matrix result = {a.rows, a.cols};
for (int i = 0; i < result.rows; i++) {
for (int j = 0; j < result.cols; j++) {
result.data[i][j] = num * a.data[i][j];
}
}
return result;
}
// 矩阵转置
Matrix transpose(Matrix a) {
Matrix transposed = {a.cols, a.rows};
for (int i = 0; i < a.rows; i++) {
for (int j = 0; j < a.cols; j++) {
transposed.data[j][i] = a.data[i][j];
}
}
return transposed;
}
int main() {
// 示例用法
Matrix m1 = {3, 3, {1, 2, 3, 4, 5, 6, 7, 8, 9}};
Matrix m2 = {3, 3, {10, 11, 12, 13, 14, 15, 16, 17, 18}};
Matrix m_add = add(m1, m2);
Matrix m_subtract = subtract(m1, m2);
Matrix m_multiply = multiply(m1, m2); // 假设m1和m2可相乘
Matrix m_num = numMultiply(2, m1);
Matrix m_transpose = transpose(m1);
// 打印结果矩阵
// ...
return 0;
}
```
阅读全文
相关推荐

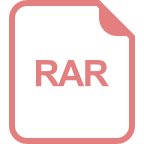
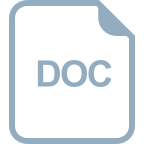
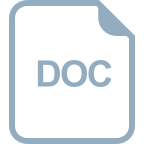
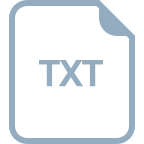
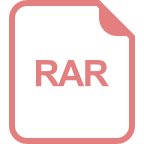
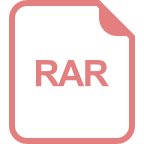
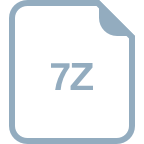
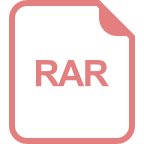
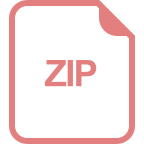
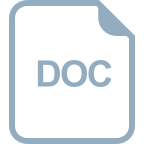
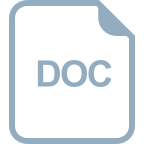
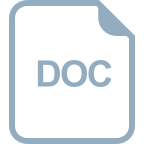
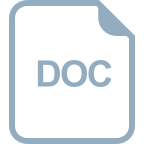
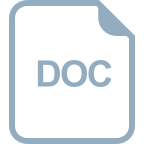