如何在Java中使用Apache HttpClient库发送包含JSON数据的POST请求,并确保数据通过UTF-8编码正确发送?请结合代码示例进行说明。
时间: 2024-12-21 14:12:51 浏览: 7
为了掌握在Java中使用Apache HttpClient库发送包含JSON数据的POST请求,并确保数据通过UTF-8编码正确发送,你需要了解几个关键步骤。首先,确保你已经安装并导入了Apache HttpClient库及其相关组件。接着,按照以下步骤构建你的POST请求:
参考资源链接:[使用Httpclient模拟POST请求:JSON封装与表单数据示例](https://wenku.csdn.net/doc/6412b69cbe7fbd1778d47578?spm=1055.2569.3001.10343)
1. 创建HttpPost实例,传入目标URL。例如:
```java
HttpPost httpPost = new HttpPost(
参考资源链接:[使用Httpclient模拟POST请求:JSON封装与表单数据示例](https://wenku.csdn.net/doc/6412b69cbe7fbd1778d47578?spm=1055.2569.3001.10343)
相关问题
Apache HttpClient java库中,发送请求怎样携带json数据?
在Apache HttpClient库中,发送请求携带JSON数据有两种方式:
1. 使用StringEntity
可以使用StringEntity将JSON数据转换为字符串,然后将其设置为请求实体。示例代码如下:
```
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
httpPost.setHeader("Content-type", "application/json");
StringEntity stringEntity = new StringEntity(jsonData, ContentType.APPLICATION_JSON);
httpPost.setEntity(stringEntity);
CloseableHttpResponse httpResponse = httpClient.execute(httpPost);
```
其中,jsonData是一个包含JSON数据的字符串。
2. 使用JSONObject
另一种方法是使用JSONObject库将JSON数据转换为Java对象,然后将其转换为字符串,最后将其设置为请求实体。示例代码如下:
```
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
httpPost.setHeader("Content-type", "application/json");
JSONObject jsonObject = new JSONObject();
jsonObject.put("key1", "value1");
jsonObject.put("key2", "value2");
StringEntity stringEntity = new StringEntity(jsonObject.toString(), ContentType.APPLICATION_JSON);
httpPost.setEntity(stringEntity);
CloseableHttpResponse httpResponse = httpClient.execute(httpPost);
```
其中,jsonObject是一个包含JSON数据的Java对象。
如何在Java中发送POST请求并将入参作为JSON数据?
在Java中,发送POST请求并携带JSON数据通常需要使用网络请求库,如`HttpURLConnection`、`Apache HttpClient` 或者第三方库如`Spring Framework`的`RestTemplate` 或 `OkHttp`。这里是一个使用`RestTemplate`的例子:
```java
import org.springframework.http.*;
import org.springframework.web.client.RestTemplate;
import com.fasterxml.jackson.databind.ObjectMapper; // 如果你的JSON对象是自定义类,需要这个库来进行序列化
public class Main {
private static final String ENDPOINT = "https://example.com/api"; // API 接口地址
public static void main(String[] args) {
// 创建一个JSON对象或自定义的Pojo对象
MyJsonData jsonData = new MyJsonData(); // 替换成你自己的类名
jsonData.setField1("value1");
jsonData.setField2("value2");
// 将对象转换为JSON字符串
ObjectMapper mapper = new ObjectMapper();
String jsonString = mapper.writeValueAsString(jsonData);
// 使用RestTemplate发送POST请求
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
HttpEntity<String> request = new HttpEntity<>(jsonString, headers);
RestTemplate restTemplate = new RestTemplate();
try {
ResponseEntity<String> response = restTemplate.postForEntity(ENDPOINT, request, String.class);
if (response.getStatusCode().is2xxSuccessful()) {
String responseBody = response.getBody();
System.out.println("Response: " + responseBody);
} else {
System.out.println("Error: " + response.getStatusCode() + ", " + response.getResponseBody());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
// 自定义类MyJsonData
class MyJsonData {
private String field1;
private String field2;
// getters and setters...
}
```
在这个例子中,我们首先创建一个`MyJsonData`对象,然后用`ObjectMapper`将其转换为JSON字符串。然后设置`Content-Type`为`application/json`,并构造`HttpEntity`用于POST请求。`RestTemplate`的`postForEntity`方法会处理整个过程。
阅读全文
相关推荐
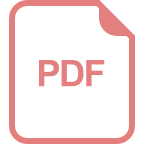
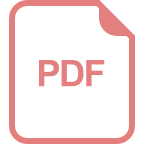
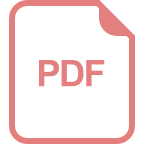













