ctypes.CDLL
时间: 2024-09-21 22:03:47 浏览: 22
`ctypes.CDLL` 是 Python 的 `ctypes` 模块提供的一种功能,它允许开发者动态链接到共享库(也称为动态链接库或 DLL)。在 Python 中,当我们需要使用 C 或 C++ 编写的库函数时,`CDLL` 就派上用场了。
`ctypes.CDLL` 是用来加载和调用操作系统特定的二进制文件(通常是 .dll 文件在 Windows 上,或 .so 文件在 Linux 和 macOS 上),这个过程通常涉及到底层的内存管理和函数指针操作。通过创建 `CDLL` 对象并指定库的路径,你可以访问其中的函数、全局变量等,并像调用普通的 Python 函数一样使用它们。
例如:
```python
import ctypes
# 加载名为 'mylib.dll' 的动态库
my_library = ctypes.CDLL('path_to_mylib.dll')
# 获取库中某个函数的地址
func_address = my_library.my_function_address
# 调用该函数,传入参数
result = func_address(42, "Hello")
```
相关问题
python指令ctypes.CDLL
`ctypes.CDLL` 是 Python 的 `ctypes` 模块中的一个功能,它用于动态链接和加载共享库(通常称为动态链接库,或 DLL 文件)在 Python 程序中。`CDLL` 类允许你像操作本地 C 函数一样调用外部库的函数,这对于需要使用 C 或 C++ 编写的库,并且不希望直接编写 C 扩展模块的情况下非常有用。
当你使用 `ctypes.CDLL` 时,你需要提供库文件的路径,例如:
```python
import ctypes
# 加载名为 "mylibrary.dll" 的库
my_library = ctypes.CDLL("path/to/mylibrary.dll")
# 调用库中的函数
function_name = my_library.my_function
function_name.argtypes = [ctypes.c_int, ctypes.c_char_p] # 设置函数参数类型
result = function_name(42, b"Hello") # 调用并传递参数
```
在这个例子中,`my_function` 是库中定义的一个函数,`argtypes` 属性设置参数类型,以便正确地解释传递给函数的数据。
ctypes.CDLL()
`ctypes.CDLL()` 是 Python 中的一个函数,用于加载动态链接库(DLL)并创建一个可调用的对象,以便在 Python 中调用其中定义的函数。
`ctypes.CDLL()` 的基本语法如下:
```python
ctypes.CDLL(name, mode=ctypes.DEFAULT_MODE, handle=None, use_errno=False, use_last_error=False)
```
- `name`:要加载的 DLL 的文件名或路径。
- `mode`:加载模式,默认为 `ctypes.DEFAULT_MODE`。
- `handle`:要使用的现有句柄。如果提供了句柄,则忽略 `name` 和 `mode`。
- `use_errno`:如果为 `True`,则将 `ctypes.get_errno()` 设置为标准 C 函数调用失败时的错误代码。
- `use_last_error`:如果为 `True`,则将 `ctypes.get_last_error()` 设置为标准 C 函数调用失败时的错误代码。
`ctypes.CDLL()` 将返回一个可调用的对象,可以使用该对象调用 DLL 中定义的函数。
以下是一个示例,演示如何使用 `ctypes.CDLL()` 加载 DLL 并调用其中的功能:
```python
import ctypes
# 加载 DLL
my_dll = ctypes.CDLL("my_dll.dll")
# 调用 DLL 中的函数
result = my_dll.my_function()
# 打印结果
print(result)
```
在上面的示例中,我们加载了名为 "my_dll.dll" 的 DLL,并调用了其中的 "my_function()" 函数。然后,我们打印了函数的返回结果。
问题产生的原因:
`ctypes.CDLL()` 主要用于与 C 或其他语言编写的动态链接库进行交互。它提供了一种通过加载 DLL 并创建可调用对象来调用其中定义的函数的简单方法。
相关推荐
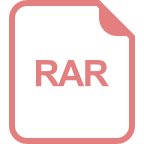
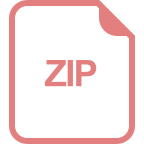
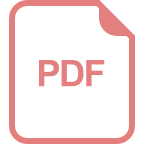












