用C语言解决:循环队列的入队出队
时间: 2024-11-05 09:26:58 浏览: 28
在C语言中,我们可以使用数组来实现循环队列,因为它支持索引超出边界的情况。这里是一个简单的循环队列的结构定义以及入队(enqueue)和出队(dequeue)操作的实现:
```c
#include <stdio.h>
#define MAX_SIZE 10 // 定义队列的最大容量
typedef struct {
int data[MAX_SIZE]; // 存储数据的数组
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = q->rear = -1;
}
// 入队操作 (enqueue)
void enqueue(Queue *q, int value) {
if ((q->rear + 1) % MAX_SIZE == q->front) { // 判满
printf("Queue is full.\n");
return;
}
q->rear = (q->rear + 1) % MAX_SIZE; // 循环更新队尾
q->data[q->rear] = value;
printf("Enqueued %d\n", value);
}
// 出队操作 (dequeue)
int dequeue(Queue *q) {
if (q->front == -1) { // 空队列
printf("Queue is empty.\n");
return -1; // 返回空值或错误码
}
int value = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE; // 更新队头
return value;
}
int main() {
Queue queue;
initQueue(&queue);
enqueue(&queue, 1);
enqueue(&queue, 2);
enqueue(&queue, 3); // 示例操作
int removed = dequeue(&queue);
printf("Removed: %d\n", removed);
return 0;
}
```
在这个例子中,我们首先检查队列是否已满(`rear`接近`front`),然后进行元素的添加。出队时,我们会检查队列是否为空,然后移除并返回队首元素。
阅读全文
相关推荐
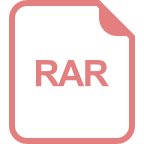
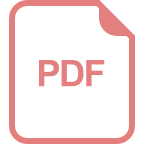
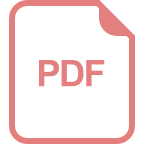
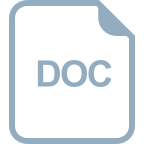
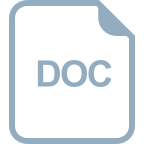

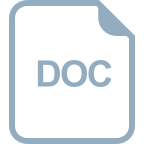
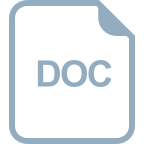









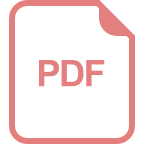
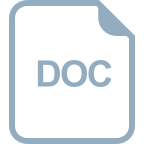