计算机图形openGL画圆
时间: 2023-11-24 15:46:29 浏览: 35
在OpenGl中,绘制圆可以通过绘制多边形来实现,多边形的边数越多,圆的轮廓就越接近于圆形。以下是基于OpenGL的C++代码示例,可以绘制一个近似圆形:
```
void drawCircle(float radius, int num_segments) {
glBegin(GL_LINE_LOOP);
for (int i = 0; i < num_segments; i++) {
float theta = 2.0f * 3.1415926f * float(i) / float(num_segments);//get the current angle
float x = radius * cosf(theta);//calculate the x component
float y = radius * sinf(theta);//calculate the y component
glVertex2f(x, y);//output vertex
}
glEnd();
}
```
其中,radius是圆的半径,num_segments是圆上线段的数量,如果num_segments越大,则表示圆形越精确。
相关问题
计算机图形学 opengl csdn
计算机图形学是一门研究如何利用计算机来生成和处理图形的学科,而OpenGL则是一种用于图形渲染的开放式跨平台图形库。CSND是中国最大的IT社区,拥有大量专业的计算机图形学和OpenGL技术文章和资源。
计算机图形学涉及到很多领域,比如三维建模、渲染、动画、虚拟现实等。OpenGL作为一种跨平台的图形库,可以在各种操作系统上运行,比如Windows、Linux、MacOS等。它提供了丰富的API,方便开发者进行图形渲染和处理。
在CSDN上,有很多关于计算机图形学和OpenGL的技术文章和教程,比如渲染管线的详细讲解、基本图形绘制的方法、光照和材质的处理等。这些资源能够帮助开发者更好地理解计算机图形学的原理和OpenGL的使用方法。
CSND上也有很多计算机图形学和OpenGL的学习路线和资源推荐,让想要学习这方面知识的人可以更加系统地学习相关知识。此外,CSND还有众多的社区和论坛,可以让开发者们进行交流和讨论,分享他们的学习和工作经验。
总的来说,计算机图形学和OpenGL是一门十分重要的学科和技术,而CSND作为一个IT领域的专业社区,为想要学习和了解这方面知识的人提供了丰富的资源和交流平台。希望更多的人可以通过CSND来学习和提升自己在计算机图形学和OpenGL方面的技术水平。
计算机图形学opengl Python
OpenGL是一种跨平台的图形库,用于编写2D和3D图形应用程序。它提供了一组API,用于在屏幕上呈现图形。OpenGL支持各种功能,如模型转换、光照、渲染、纹理映射等,这些功能可以帮助程序员创建各种复杂的视觉效果。
在Python中,有一个称为PyOpenGL的OpenGL绑定库,可以用于Python编程语言中的图形编程。PyOpenGL提供了Python语言与OpenGL API之间的接口,使得开发者可以使用Python进行OpenGL编程。PyOpenGL具有良好的跨平台性能和易于使用的API,使得Python成为了一个非常流行的图形编程语言。
如果您对计算机图形学和OpenGL感兴趣,建议先学习一些计算机图形学和OpenGL基础知识,然后再学习PyOpenGL。一些好的资源包括官方文档和在线教程等。
相关推荐
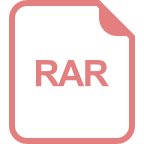
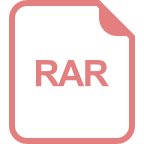
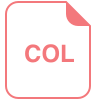
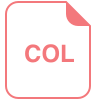
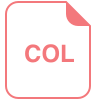
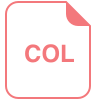
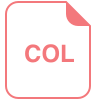







