java AEAD_AES_256_GCM解密代码
时间: 2024-10-18 14:19:57 浏览: 37
在Java中,如果你想要使用AEAD(Authenticated Encryption with Additional Data)模式如AES-256-GCM进行解密,你需要使用`javax.crypto.Cipher`类,配合`javax.crypto.spec.GCMParameterSpec`和`javax.crypto.spec.SecretKeySpec`。这里是一个基本的示例:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.GCMParameterSpec;
import javax.crypto.spec.SecretKeySpec;
public class AESGCMDecryptionExample {
private static final String SECRET_KEY = "your_secret_key_32_bytes";
private static final byte[] IV = "your_initialization_vector_12_bytes".getBytes(); // 必须与加密时使用的IV匹配
public static void main(String[] args) {
try {
// 将密钥从字符串转换为SecretKeySpec
SecretKeySpec secretKey = new SecretKeySpec(SECRET_KEY.getBytes(), "AES");
// 创建GCM参数规格
GCMParameterSpec gcmParams = new GCMParameterSpec(128, IV); // GCM的tag长度通常是128位,IV必须一次性提供
// 初始化Cipher实例并选择解密模式(ENCRYPT_MODE的相反)
Cipher cipher = Cipher.getInstance("AES/GCM/NoPadding"); // NoPadding是因为我们不需要填充
cipher.init(Cipher.DECRYPT_MODE, secretKey, gcmParams);
// 待解密的数据,包括原始数据和附加认证标签(通常在加密后的数据末尾)
byte[] encryptedDataWithTag = ...; // 获取到的实际加密内容
int tagOffset = encryptedDataWithTag.length - 16; // 假设标签占16字节
byte[] decryptedData = new byte[encryptedDataWithTag.length - tagOffset];
cipher.update(encryptedDataWithTag, 0, encryptedDataWithTag.length - tagOffset, decryptedData);
byte[] authenticationTag = new byte[tagOffset]; // 提取认证标签
System.arraycopy(encryptedDataWithTag, tagOffset, authenticationTag, 0, tagOffset);
// 验证解密结果
if (cipher.doFinal(authenticationTag) == 0) { // 如果doFinal返回0表示解密成功并且验证通过
// 解密后的数据现在在decryptedData数组中
System.out.println("Decrypted data: " + new String(decryptedData));
} else {
throw new RuntimeException("Invalid authentication tag or incorrect key.");
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
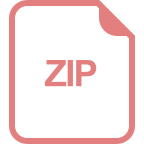
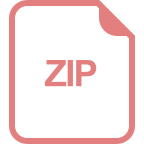
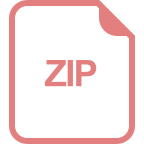

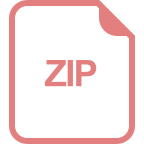
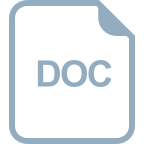
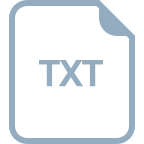
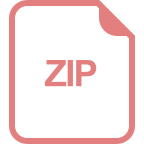
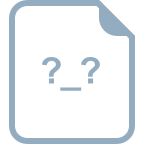
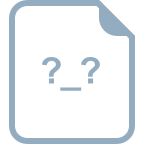
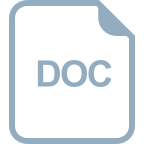
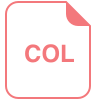





