10万元存银行,每年利息3.75%,每年计算一次利息,将本金加上利息作为第二年的本金,求n年后该账户有多少钱。 编写程序,要求:输入整数n(表示n年后),输出n年后的账户钱数(保留两位小数)。
时间: 2024-09-16 21:04:16 浏览: 44
这是一个复利计算的问题。我们可以编写一个简单的Python程序来解决这个问题:
```python
def compound_interest(principal, rate, time):
future_value = principal * (1 + rate) ** time
return round(future_value, 2)
def main():
principal = 100000 # 10万元初始本金
annual_rate = 0.0375 / 100 # 年利率转换为小数形式
time_years = int(input("请输入n年后:"))
final_amount = compound_interest(principal, annual_rate, time_years)
print(f"n年后账户的钱数为:{final_amount}元")
if __name__ == "__main__":
main()
```
在这个程序中,`compound_interest`函数根据公式 `FV = P * (1 + r)^t` 计算未来值,其中 FV 是未来的金额(包括本金和利息),P 是本金,r 是年利率,t 是时间(年)。`round` 函数用于保留两位小数。
用户输入 n 后,程序会计算出 n 年后账户的总金额并显示结果。
相关问题
用c++编写一个能计算银行存款的小程序。10万元存银行,每年利息3.75%,每年计算一次利息,将本金加上利息作为第二年的本金,求n年后该账户有多少编写程序,要求:输入整数n(表示n年后),输出n年后的账户钱数(保留两位小数)
你可以使用C++编写如下的简单银行存款计算器程序。这个程序会根据用户输入的年份n以及初始存款金额和年利率来计算最终的账户余额。
```cpp
#include <iostream>
#include <iomanip>
double calculate_balance(int n, double principal, double interest_rate) {
double balance = principal;
for (int year = 1; year <= n; ++year) {
balance += balance * interest_rate;
}
return round(balance * 100.0) / 100.0; // 四舍五入到两位小数
}
int main() {
int years_to_deposit;
std::cout << "请输入要存款的年数(n): ";
std::cin >> years_to_deposit;
double initial_deposit = 100000.0; // 初始存款10万
double annual_interest_rate = 0.0375; // 年利率3.75%
double final_balance = calculate_balance(years_to_deposit, initial_deposit, annual_interest_rate);
std::cout << "存款" << years_to_deposit << "年后,账户总额为: " << std::fixed << std::setprecision(2) << final_balance << "元" << std::endl;
return 0;
}
```
在这个程序中,`calculate_balance`函数负责核心计算,它通过一个循环逐年累加利息并更新总金额。最后,在`main`函数中获取用户的输入,并显示结果。
C++亮亮把n元按照m年期整存存入银行,按照目前银行的年利率,请问到期后亮亮可以连本带息总共拿到多少钱?(6.1.98) 存期(整存整取) 年利率 1年 3.25% 2年 3.75% 3年~4年 4.25% 5年及5年以上 4.75%
根据题目中的数据,我们可以写出以下的代码:
```c++
#include <iostream>
using namespace std;
int main() {
int n, m;
double rate, money;
cout << "请输入存款金额和存款期限(单位:年):";
cin >> n >> m;
if (m == 1) {
rate = 0.0325;
} else if (m == 2) {
rate = 0.0375;
} else if (m >= 3 && m <= 4) {
rate = 0.0425;
} else {
rate = 0.0475;
}
money = n * (1 + rate * m);
cout << "到期后可以拿到 " << money << " 元。" << endl;
return 0;
}
```
这段代码中,我们先输入存款金额 `n` 和存款期限 `m`,然后根据不同的存款期限选择不同的年利率 `rate`,最后计算出到期后可以拿到的金额 `money`。最终输出结果。
相关推荐
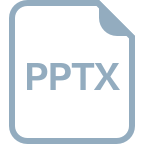
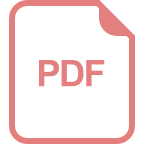
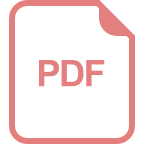













