c++ 用 activemq-cpp 实现上传数据功能
时间: 2024-04-12 15:28:10 浏览: 159
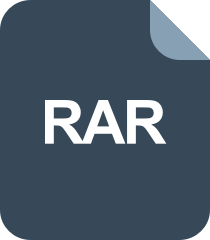
activeMQ在文件上传的应用
要使用ActiveMQ-CPP库实现上传数据功能,你需要按照以下步骤进行操作:
1. 首先,确保你已经安装了ActiveMQ-CPP库。你可以在ActiveMQ官方网站上找到相应的下载链接和安装说明。
2. 在你的C++项目中,包含ActiveMQ-CPP的头文件:
```cpp
#include <activemq/core/ActiveMQConnectionFactory.h>
#include <activemq/library/ActiveMQCPP.h>
```
3. 创建一个连接工厂,并设置ActiveMQ服务器的连接地址和端口:
```cpp
std::string brokerURI = "tcp://localhost:61616";
activemq::core::ActiveMQConnectionFactory connectionFactory(brokerURI);
```
4. 创建一个连接,并启动它:
```cpp
activemq::core::Connection* connection = connectionFactory.createConnection();
connection->start();
```
5. 创建一个会话,并指定会话类型和订阅目的地:
```cpp
activemq::core::Session* session = connection->createSession(activemq::core::Session::AUTO_ACKNOWLEDGE);
activemq::core::Destination* destination = session->createQueue("your_queue_name");
```
6. 创建一个消息生产者,用于发送数据:
```cpp
activemq::core::MessageProducer* producer = session->createProducer(destination);
```
7. 构建一个消息对象,并设置消息内容:
```cpp
activemq::core::TextMessage* message = session->createTextMessage();
message->setText("Your data here");
```
8. 发送消息到目的地:
```cpp
producer->send(message);
```
9. 清理资源并关闭连接:
```cpp
delete message;
delete producer;
session->close();
connection->close();
```
这只是一个基本的示例,你可以根据你的需求进行进一步的代码编写和功能实现。请确保在使用完资源后正确释放它们,以避免内存泄漏。
希望这个示例能帮到你,如果你还有其他问题,请随时提问!
阅读全文
相关推荐
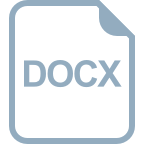
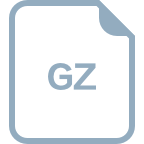

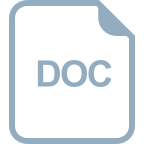

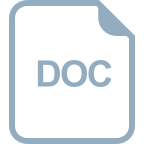
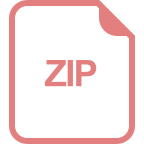
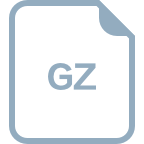
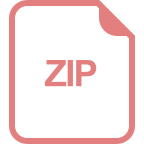
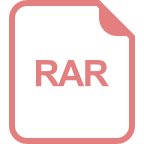
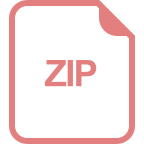
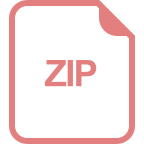
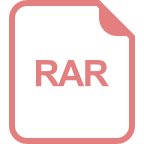
