上述代码改为c++的实现
时间: 2024-04-15 12:27:14 浏览: 20
以下是一个示例的 C++ 实现,使用 ActiveMQ 进行通信,并添加重连机制:
```cpp
#include <activemq/core/ActiveMQConnectionFactory.h>
#include <activemq/library/ActiveMQCPP.h>
#include <cms/Connection.h>
#include <cms/Session.h>
#include <cms/ExceptionListener.h>
#include <cms/Exception.h>
using namespace activemq::core;
using namespace cms;
// 最大重连尝试次数
const int MAX_RECONNECT_ATTEMPTS = 3;
// 重连延迟时间(毫秒)
const int RECONNECT_DELAY = 5000;
class MyExceptionListener : public ExceptionListener {
public:
MyExceptionListener(Connection* connection) : connection(connection) {}
virtual void onException(const CMSException& ex) override {
std::cout << "Connection exception: " << ex.getMessage() << std::endl;
int reconnectCount = 0;
while (!connection->isStarted() && reconnectCount < MAX_RECONNECT_ATTEMPTS) {
try {
std::cout << "Reconnecting..." << std::endl;
connection->start();
} catch (CMSException& e) {
std::cout << "Failed to reconnect: " << e.getMessage() << std::endl;
}
reconnectCount++;
if (connection->isStarted()) {
std::cout << "Reconnected successfully!" << std::endl;
} else {
std::cout << "Failed to reconnect!" << std::endl;
::sleep(RECONNECT_DELAY / 1000);
}
}
}
private:
Connection* connection;
};
int main() {
// 设置 ActiveMQ broker 地址
std::string brokerURI = "tcp://localhost:61616";
// 设置 Topic 名称
std::string topicName = "topicName";
try {
// 初始化 ActiveMQ C++ 库
activemq::library::ActiveMQCPP::initializeLibrary();
// 创建连接工厂
ActiveMQConnectionFactory* connectionFactory = new ActiveMQConnectionFactory(brokerURI);
// 创建连接
Connection* connection = connectionFactory->createConnection();
// 创建异常监听器
MyExceptionListener* exceptionListener = new MyExceptionListener(connection);
connection->setExceptionListener(exceptionListener);
// 启动连接
connection->start();
// 创建会话
Session* session = connection->createSession(Session::AUTO_ACKNOWLEDGE);
// 创建目标(Topic)
Destination* destination = session->createTopic(topicName);
// 创建消息生产者
MessageProducer* producer = session->createProducer(destination);
// 创建消息
TextMessage* message = session->createTextMessage("Hello, ActiveMQ!");
// 发送消息
producer->send(message);
// 关闭连接
connection->close();
// 清理资源
delete message;
delete producer;
delete destination;
delete session;
delete connectionFactory;
// 终止 ActiveMQ C++ 库
activemq::library::ActiveMQCPP::shutdownLibrary();
} catch (CMSException& ex) {
std::cout << "Exception occurred: " << ex.getMessage() << std::endl;
}
return 0;
}
```
在上述代码中,我们创建了一个自定义的异常监听器 `MyExceptionListener`,并将其设置为连接的异常监听器。在异常监听器的回调函数中,我们使用 while 循环来尝试重新启动连接,直到连接成功或达到最大重连尝试次数。在重连过程中,我们使用 `::sleep` 函数来添加延迟等待时间。
通过添加重连机制,当连接断开或出现异常时,程序可以自动进行重连,以确保消息的可靠传输和处理。根据具体需求,还可以根据实际情况进行适当的错误处理和日志记录。
相关推荐
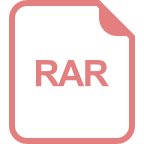
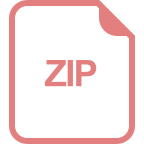
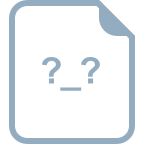














