用 AcTiveMQ-cpp3.7.0库 c++ 实现一个简单得上传功能
时间: 2024-04-10 10:35:04 浏览: 33
要使用 ActiveMQ-CPP 3.7.0 库实现一个简单的上传功能,你可以按照以下步骤进行:
1. 安装 ActiveMQ-CPP 库:首先,从 ActiveMQ-CPP 的官方网站或其他可靠来源下载并安装 ActiveMQ-CPP 3.7.0 库。
2. 包含头文件:在你的 C++ 代码中,包含 ActiveMQ-CPP 的头文件,例如:
```cpp
#include <activemq/core/ActiveMQConnectionFactory.h>
#include <activemq/library/ActiveMQCPP.h>
```
3. 初始化 ActiveMQ-CPP 库:在你的代码中添加初始化 ActiveMQ-CPP 库的语句,例如:
```cpp
activemq::library::ActiveMQCPP::initializeLibrary();
```
4. 创建连接工厂:创建 ActiveMQ 连接工厂对象,并设置连接 ActiveMQ 服务器所需的参数,例如:
```cpp
const std::string brokerURI = "tcp://localhost:61616";
activemq::core::ActiveMQConnectionFactory factory(brokerURI);
```
5. 创建连接和会话:使用连接工厂创建连接和会话对象,例如:
```cpp
activemq::core::Connection* connection = factory.createConnection();
connection->start();
activemq::core::Session* session = connection->createSession();
```
6. 创建消息生产者:创建消息生产者对象,用于发送上传的消息,例如:
```cpp
activemq::core::Destination* destination = session->createQueue("uploadQueue");
activemq::core::MessageProducer* producer = session->createProducer(destination);
```
7. 创建消息和发送:根据你的需求,创建消息对象并发送到 ActiveMQ 服务器,例如:
```cpp
std::string filePath = "path/to/file";
std::string fileName = "example.txt";
std::ifstream file(filePath, std::ios::binary);
std::vector<char> buffer(std::istreambuf_iterator<char>(file), {});
activemq::core::BytesMessage* message = session->createBytesMessage();
message->setReadOnlyBody(false);
message->writeBytes(&buffer[0], buffer.size());
message->setStringProperty("FileName", fileName);
producer->send(message);
```
8. 清理和关闭连接:在上传完成后,确保关闭连接和释放资源,例如:
```cpp
producer->close();
session->close();
connection->close();
delete producer;
delete session;
delete connection;
activemq::library::ActiveMQCPP::shutdownLibrary();
```
上述步骤仅提供了一个基本的上传功能的示例,你可以根据你的具体需求进行修改和扩展。同时,还需要根据你的 ActiveMQ 服务器的配置和需求进行相应的调整。请确保在使用 ActiveMQ-CPP 库时遵循其官方文档和最佳实践。
相关推荐
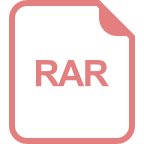
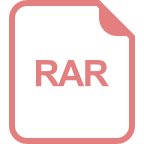














