混沌系统python
时间: 2023-11-01 08:58:14 浏览: 69
混沌系统是指一类在动力学上表现出极其敏感依赖于初始条件的系统。在Python中,可以使用一些数学库(如NumPy和Matplotlib)来模拟和可视化混沌系统。
混沌系统的一个典型例子是Logistic方程。Logistic方程是一个描述人口增长的模型,其动力学特征呈现出复杂的非线性行为。下面是一个用Python绘制Logistic方程动画的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
def func(t_list, m):
x = 0.8
x_list = []
for j in t_list:
x = m * x * (1 - x)
x_list.append(x)
return x_list
if __name__ == '__main__':
m_list = [0.2, 0.9, 1.2, 2.7, 3.2, 3.5, 3.6, 4]
t_list = np.linspace(1, 100, num=100)
for m in m_list:
x_list = func(t_list, m)
plt.cla()
plt.plot(t_list, x_list)
plt.title('Logistic m = ' + str(m))
plt.xlabel('t')
plt.ylabel('x(t)')
plt.xlim((0, 100))
plt.ylim((0, 1))
plt.pause(3)
```
这段代码会生成一个动画,展示了不同m值下Logistic方程的演化过程。在动画中,x轴代表时间,y轴代表系统的状态值。
另一个常见的混沌系统是Mandelbrot二维混沌动力方程。下面是一个计算Mandelbrot集合的示例代码:
```python
import numpy as np
def mandelbrot_set(x, y, iterateCount):
c = np.array(x + 1j * y)
z = np.zeros(c.shape, dtype=complex)
result = np.ones(c.shape)
m = np.ones(c.shape, dtype=bool)
for i in range(iterateCount):
z[m] = z[m] ** 2 + c[m]
m = np.abs(z) < escapeRadius
result[m] = i
return result
```
这段代码中,使用了NumPy库来进行矩阵运算,通过迭代计算得到Mandelbrot集合的图像。
相关推荐
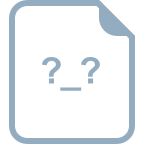
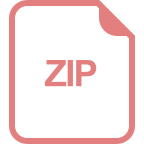














