pygame实现小球碰撞
时间: 2024-03-18 09:37:07 浏览: 204
Pygame是一个用于开发2D游戏的Python库,可以实现小球碰撞效果。下面是一个简单的示例代码来演示如何使用Pygame实现小球碰撞:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口大小和标题
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Ball Collision")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 定义小球类
class Ball:
def __init__(self, x, y, radius, color):
self.x = x
self.y = y
self.radius = radius
self.color = color
self.dx = random.randint(-5, 5) # 随机生成小球的水平速度
self.dy = random.randint(-5, 5) # 随机生成小球的垂直速度
def move(self):
self.x += self.dx
self.y += self.dy
def draw(self):
pygame.draw.circle(screen, self.color, (self.x, self.y), self.radius)
def check_collision(self, other_ball):
distance = ((self.x - other_ball.x) ** 2 + (self.y - other_ball.y) ** 2) ** 0.5
if distance <= self.radius + other_ball.radius:
return True
else:
return False
# 创建小球对象列表
balls = []
for _ in range(10):
x = random.randint(0, width)
y = random.randint(0, height)
radius = random.randint(10, 50)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
ball = Ball(x, y, radius, color)
balls.append(ball)
# 游戏主循环
running = True
clock = pygame.time.Clock()
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill(BLACK)
# 移动和绘制小球
for ball in balls:
ball.move()
ball.draw()
# 检测小球碰撞
for i in range(len(balls)):
for j in range(i + 1, len(balls)):
if balls[i].check_collision(balls[j]):
balls[i].dx *= -1
balls[i].dy *= -1
balls[j].dx *= -1
balls[j].dy *= -1
pygame.display.flip()
clock.tick(60)
# 退出游戏
pygame.quit()
```
这段代码创建了一个窗口,生成了一些随机大小和颜色的小球,并且让它们在窗口中移动。当两个小球碰撞时,它们的速度会反向改变,从而实现了小球的碰撞效果。
阅读全文
相关推荐
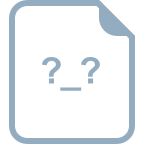
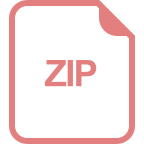
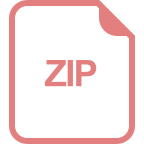



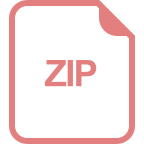
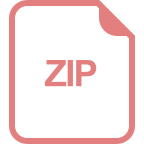
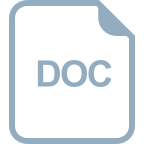
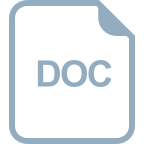
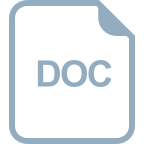


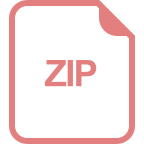
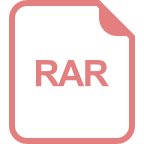
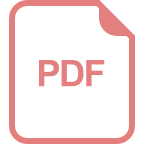
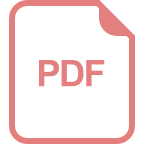
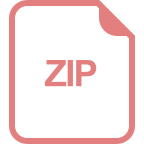
